While its initial purpose was to serve as a scripting language for front-end web applications, JavaScript has continually evolved to establish an almost omnipresent presence in software development. The JavaScript history reveals a journey of constant innovation and adaptation, making it hardly surprising that JavaScript reigns supreme as the most utilized language among GitHub users, the world’s largest software development and collaboration platform.
GitHub has now become the go-to hub for sharing code and open-source projects, boasting a plethora of repositories dedicated to JavaScript. This article will delve into some of the most outstanding open-source JavaScript projects found on GitHub.
Is JavaScript Open-source?
JavaScript itself isn't open-source, as it's a standardized programming language maintained by an organization called ECMA International. However, there are countless open-source JavaScript projects available on various websites for developers to explore, contribute to, and learn from.
Whether you're a seasoned coder or just starting, getting involved with these projects can be a great way to enhance your skills. Contributing to these projects aligns with web development trends, helping developers stay updated with the latest tools and technologies. Many developers begin their journey by contributing to the best open-source projects for JavaScript, gaining hands-on experience while also helping to improve the tools and libraries that the coding community relies on.
Nest
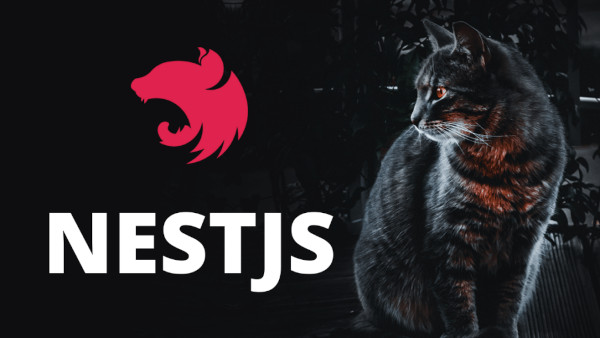
NestJS is a popular open-source JavaScript framework for building scalable and maintainable server-side applications. It is often described as a progressive framework because it is built on top of Node.js and TypeScript, and is designed to be flexible and adaptable to various use cases, from building RESTful APIs to full-featured web applications and microservices. Interestingly, NestJS draws inspiration from Angular's structure and approach, making it particularly advantageous if you hire Angular JS developers who are already familiar with similar design patterns. This connection also complements the new features in Angular 17, improving development efficiency.
Here are some key features and aspects of NestJS:
- TypeScript Support. NestJS is primarily written in TypeScript and encourages developers to use TypeScript for building applications. TypeScript provides strong typing and helps catch errors at compile time, enhancing code quality and developer productivity. In case you need to hire typescript developers, MaybeWorks is ready to provide you with top-level engineers.
- Express.js Integration. NestJS is developed on top of the popular Express.js framework for handling HTTP requests and routing. It provides an abstraction layer over Express, making it more structured and adding additional features. A great alternative to Express.js is the Fastify framework.
- WebSockets Support. NestJS includes built-in support for WebSockets, making it suitable for real-time applications that require bidirectional communication between the server and clients. We also recommend you read our article about WebSockets pros and cons.
- CLI Tool. NestJS provides a command-line interface (CLI) tool that helps in generating boilerplate code, modules, controllers, and other components to kickstart your application development.
- Numerous solutions. NetJS offers a wealth of solutions contributed by both NestJS developers and the thriving community, providing many resources and tools to cater to various needs.
NestJS is well-suited for building scalable, maintainable, and structured back-end applications, especially when working with TypeScript. It has gained popularity for its developer-friendly features and the ability to build from robust APIs to microservices. What is microservices? It’s an approach that NestJS supports, making it easier to scale, maintain, and deploy applications efficiently.
Date-fns
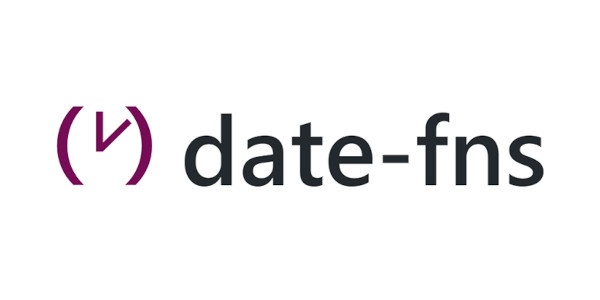
Date-fns is a popular JavaScript library used for working with dates and times. It provides a set of utility functions for parsing, formatting, manipulating, and comparing dates and times in a more developer-friendly way compared to the built-in Date object in JavaScript. Date-fns is often chosen for open-source JavaScript projects for beginners thanks to its simplicity, immutability, and consistency in handling date and time operations, making it an excellent starting point for learning and making your first contributions.
Here are some key features and aspects of Date-fns:
- Immutable. Date-fns promotes immutability, which means that operations on dates and times do not modify the original date but instead return a new date object. This helps prevent unintended side effects in your code.
- Modularity. Date-fns is modular, allowing you to import only the specific functions you need, which helps keep your bundle size small if you use it in a web application.
- Consistency. The API of date-fns is designed to be consistent and predictable. Function names are intuitive, and the library provides a wide range of functions for various date and time operations.
- Support for Different Timezones. Date-fns-tz, an extension library, extends date-fns to support working with timezones, making it easier to handle daylight saving time changes and time zone conversions.
- Parsing and Formatting. Date-fns allows you to easily parse date strings into JavaScript Date objects and format dates in various ways, including custom formatting.
- Relative Time. It provides functions for working with relative time, such as getting the difference between two dates in human-readable terms (e.g., "2 hours ago").
- Localization. Date-fns supports localization, allowing you to format dates and times according to different locales and languages.
Overall, Date-fns is a valuable tool for JavaScript developers who need to work with dates and times in their applications, providing a consistent and easy-to-use API for managing date- and time-related tasks. For CRM applications that rely on accurate date and time management, you can hire dedicated CRM software developers to integrate Date-fns seamlessly into your project.
Socket.IO
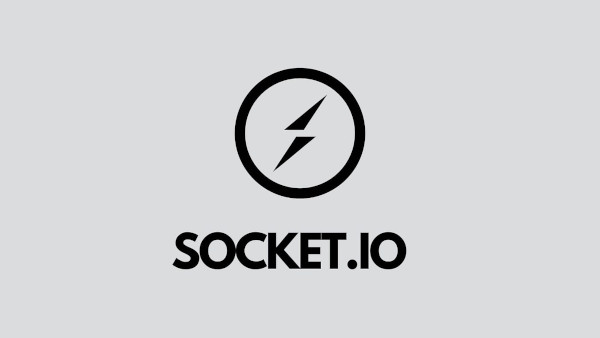
Socket.IO is a well-liked JavaScript library that allows bidirectional communication between web clients (typically browsers) and servers real-time. It is often used to build interactive web applications and online games where data needs to be pushed from the server to the client and vice versa in real time. For developers, the official website offers comprehensive resources and guides to effectively implement Socket.IO in projects.
Here are some key features and aspects of Socket.IO:
- WebSocket Support. Socket.IO primarily uses WebSockets as the underlying transport protocol for real-time communication. WebSockets allow for full-duplex communication, so data can go back and forth between the server and the client at any time without the client asking for updates.
- Fallback Mechanisms. Socket.IO includes fallback mechanisms to ensure real-time communication works even in environments where WebSocket support may be limited or restricted, such as older browsers. It can fall back to technologies like long polling, JSONP, or other transport methods when necessary.
- Event-Driven Communication. For those exploring JS open-source projects, Socket.IO offers a robust solution for implementing real-time communication. Both the client and server can emit events and listen for events, making it easy to define custom communication protocols and handle specific actions in real time.
- Rooms and Namespaces. Socket.IO allows you to organize clients into rooms or namespaces. This enables you to broadcast messages to specific groups of clients or separate different parts of your application’s real-time communication.
- Bi-directional Communication. Socket.IO supports both server-to-client (pushing data from the server to the client) and client-to-server communication. This bidirectional capability is crucial for building interactive applications.
- Cross-Browser Compatibility. Socket.IO is designed to work across various web browsers and platforms, ensuring compatibility with a wide range of devices and environments.
- Middleware Support. You can use middleware functions with Socket.IO, just like you would with popular web frameworks like Express.js. This feature allows you to add custom logic to incoming and outgoing messages, with the flexibility to integrate your own contribution to the application’s workflow.
- Scalability. Socket.IO can be used in clustered server setups and is often used with load balancing to handle many concurrent connections.
Socket.IO abstracts the complexities of working with lower-level WebSocket and long polling protocols, providing a simple and reliable API for building real-time applications. For developers building real-time applications, understanding the benefits of the Bun JavaScript runtime compared to Deno can be helpful.
Axios
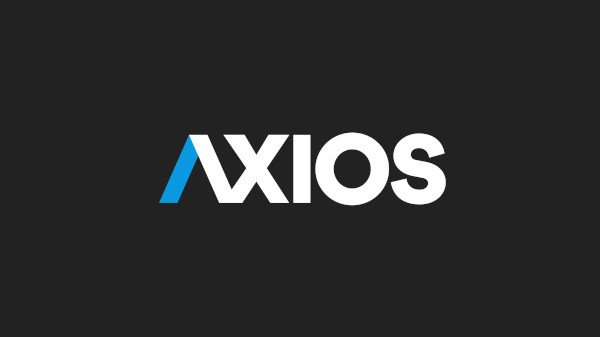
Axios is a prominent JavaScript library used to make asynchronous HTTP requests to web servers. It is commonly used in browser-based JavaScript and NodeJS app development to interact with RESTful APIs or other web services. Axios simplifies sending HTTP requests and handling responses, making it easier for developers to work with data from external sources. If you’re looking to optimize your Node.js projects, it’s a smart move to hire Node.js developers who are proficient in using tools like Axios, as well as in understanding the documentation that supports these tools.
Here are some key features and aspects of Axios:
- Promise-based. Axios is built on top of JavaScript Promises, making it comfortable to work with asynchronous operations. You can use async/await or .then() syntax to handle responses and perform actions after the request is complete.
- Supports Multiple Environments. Axios is designed to work in various environments, including browsers and Node.js. This versatility makes it a suitable choice for both client-side and server-side applications.
- HTTP Methods. Axios supports all standard HTTP methods, such as GET, POST, PUT, DELETE, etc., allowing you to perform a wide range of HTTP requests.
- Request and Response Interceptors. You can use interceptors in Axios to globally modify requests or responses prior to sending or receiving. Tasks like this can be useful for tasks like adding authentication headers or handling error responses consistently.
- Automatic JSON Parsing. Axios automatically parses JSON responses, simplifying the process of working with JSON data from API endpoints. You can also customize how the response data is processed.
- Error Handling. Axios provides built-in error handling, allowing you to catch and handle errors that may arise during the HTTP request process, such as network issues or HTTP status code errors.
- Canceling Requests. Axios supports canceling HTTP requests, which can be helpful when you need to abort a request that is no longer needed.
- Interacting with APIs. Developers often use Axios to interact with RESTful APIs or other HTTP-based web services. It’s common to use Axios to send GET requests to fetch data, POST and PUT requests to send data to a server, and DELETE requests to remove resources.
- Promise-based API. Axios provides a clean and consistent API for making requests, with chaining capabilities that make it easy to perform multiple asynchronous operations sequentially.
Axios is a widely adopted library in the JavaScript ecosystem due to its simplicity, flexibility, and ease of use when working with HTTP requests. It simplifies communicating with APIs and fetching data from web servers, making it a popular choice for those working on open-source JS projects. In a React to Next JS migration, Axios remains an excellent choice for managing HTTP requests.
ESlint
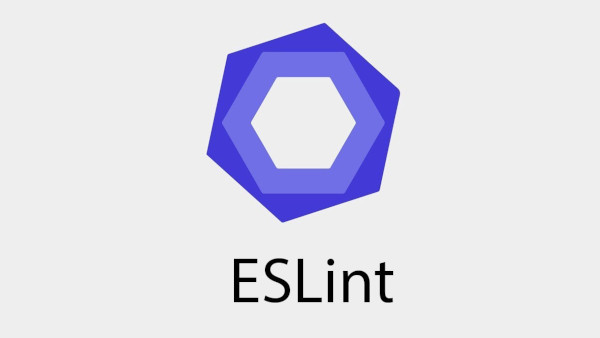
ESLint is an open-source JavaScript linting tool that helps developers identify and solve issues in their JavaScript code. Linting is the process of analyzing code to find issues related to coding style, syntax errors, potential bugs, and other programming best practices. ESLint specifically focuses on enforcing coding conventions and catching common programming mistakes. If you’re looking to maintain high code quality in your React projects, it’s a great idea to hire React.js developers who are experienced with tools like ESLint and understand how ReactJS works to manage pull requests effectively, keeping your codebase clean and error-free.
Here are some key features and aspects of ESLint:
- Customizable Rules. ESLint allows developers to define and configure a set of rules for their projects. These rules dictate coding standards and practices, such as indentation, variable naming, and more. You can customize these rules to match your team’s coding style or adhere to a specific coding standard, such as Airbnb, Google, or others.
- Plugin Support. ESLint is highly extensible and supports the use of plugins. You can extend ESLint’s functionality by adding plugins that provide additional rules and configurations for specific libraries, frameworks, or coding standards.
- Command-Line Interface (CLI). ESLint runs from the command line, making integrating into your development workflow easy. Developers can use the CLI to check their code for issues and automatically fix some.
- Integration with Editors and IDEs. ESLint integrates with popular code editors and integrated development environments (IDEs) like Visual Studio Code, IntelliJ IDEA, and others. It provides immediate feedback and suggestions as you write code.
- Community Support. ESLint has a vibrant and active community of developers who contribute to its development, maintain plugins, and share configurations. This community-driven approach ensures that ESLint stays up-to-date and adaptable to evolving JavaScript best practices.
- Continuous Integration (CI) Integration. ESLint can be integrated into your CI/CD (Continuous Integration/Continuous Deployment) pipelines, ensuring that your code is checked for issues automatically before it’s merged into your codebase.
- ESLint and TypeScript. ESLint can also be configured to work with TypeScript, a statically typed superset of JavaScript. This allows you to enforce coding standards and find issues in TypeScript code. Whenever you need to hire typescript developers, we are always ready to help.
In summary, ESLint is a powerful tool that helps JavaScript developers maintain code quality and consistency in their projects by providing a way to define, enforce, and automate coding standards and best practices.
TensorFlow.js
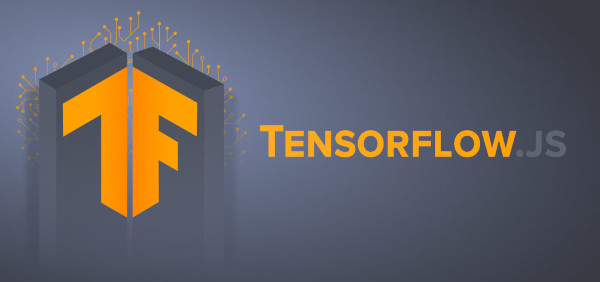
Over recent years, the surge in interest in machine learning has sparked a flurry of activity aimed at enhancing libraries to simplify the creation of machine learning models. TensorFlow.js emerges as the JavaScript counterpart to the renowned TensorFlow library, originally scripted in Python.
Through this JavaScript adaptation, the door swings open to develop and implement machine learning models within web applications, effectively extending their reach to mobile platforms. TensorFlow.js empowers developers to train machine learning models directly within the browser, alleviating the server of the computational burdens typically associated with model training. Contributions to the project can be tracked through commits in its GitHub repository, with ongoing updates and improvements managed by the project maintainers.
While this capability can be extended to mobile applications, it’s worth noting that mobile devices’ computational capabilities often fall short of demanding machine-learning applications. For those interested in exploring or contributing to open-source projects in JavaScript, there are many opportunities to engage with various tools and libraries that can help bridge these gaps.
Here are some key features and aspects of TensorFlow.js:
- Cross-Platform Compatibility. One of the standout features of TensorFlow.js is its ability to run seamlessly on both web browsers and Node.js. This cross-platform compatibility ensures that machine-learning models can be deployed in various environments. Consider working with Node.js developers who can optimize your deployment across both platforms.
- Model Training and Inference. TensorFlow.js supports both training and inference of machine learning models. Developers can use pre-trained and custom models from scratch using JavaScript, making it versatile for a wide range of applications.
- Real-Time Applications. TensorFlow.js excels in real-time applications, such as image and speech recognition, object detection, and natural language processing. It is designed to provide efficient and speedy model inference, making it ideal for applications that require rapid responses.
- Custom Model Deployment. Developers can convert pre-trained models from other TensorFlow environments (like TensorFlow Python) and deploy them using TensorFlow.js. This feature allows for reusing existing machine learning models in web-based applications.
- Extensive Compatibility. TensorFlow.js provides compatibility with a wide range of hardware, from CPUs to GPUs, and even supports WebGL for optimized graphical processing, allowing developers to leverage the full potential of available resources.
So, TensorFlow.js is a versatile and powerful JavaScript library that brings the world of machine learning to web developers. To leverage TensorFlow.js for advanced data analysis or AI features in your applications, you may want to hire JavaScript developers who are experienced with TensorFlow.js.
MeteorJS
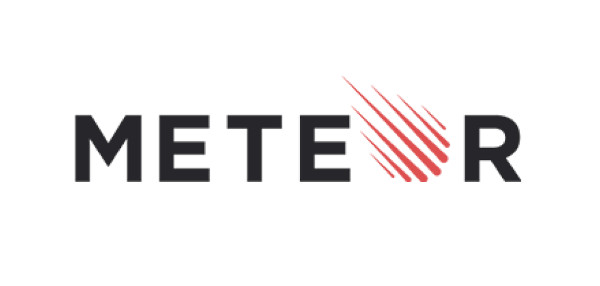
Meteor stands out as a versatile open-source platform that spans across web, mobile, and desktop environments, fostering cross-platform development. Seamlessly integrating with various other JavaScript frameworks, MeteorJS emphasizes streamlining code size, ensuring cross-platform compatibility, and facilitating smooth integration with other libraries. For those interested in open-source projects to contribute JavaScript or considering React.js alternatives, Meteor offers a valuable opportunity to engage with a dynamic and widely-used tool.
Here are some key features and aspects of MeteorJS:
- Full-Stack Development. Meteor is a full-stack platform covering both the client and server sides of development. It uses JavaScript for front-end and back-end development, simplifying the development process and minimizing the need to switch between different languages.
- Data on the Wire. Meteor follows a “data on the wire” philosophy, meaning data is automatically synchronized between the server and the client. This simplifies the development of real-time features, such as chat applications, collaborative editing, and live updates.
- Hot Code Push. Meteor allows for hot code push, which means you can install updates to your app without requiring users to refresh or reinstall the application. This feature is especially valuable for mobile apps, where updates can be delivered seamlessly.
- Data Layer. Meteor uses its own real-time database, called “Minimongo,” on the client side. On the server side, it supports a variety of databases, including MongoDB, which can be easily integrated into your application.
- Latency Compensation. Meteor compensates for network latency by allowing client-side methods to run with the assumption of instant server response. This creates a smooth user experience, even during network delays.
- Cross-Platform Compatibility. Meteor allows you to build web and mobile applications from a single codebase. It’s well-suited for creating hybrid mobile apps using technologies like Cordova.
What sets Meteor apart from its counterparts is its expansive scope that extends beyond front-end web applications. Meteor empowers developers to swiftly prototype and automatically handle data modifications. Furthermore, it offers a broad spectrum of enhancements readily accessible to cater to many use cases.
Draw.io
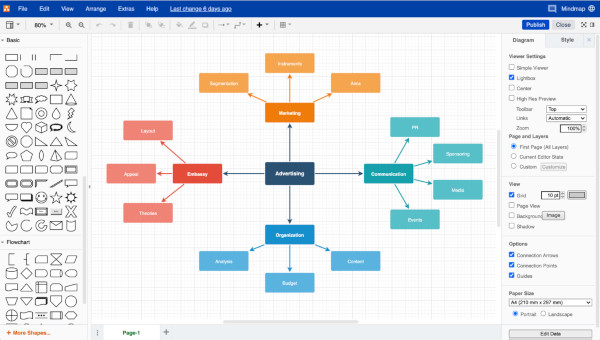
Draw.io represents a web-based diagramming platform not only improving the creation of diagrams but also offering the option to export the source code for use in other contexts. Leveraging the mxGraph library as its foundation, this versatile tool finds utility in various online drawing applications.
One of its standout features is the ability for users to store their diagrams either in the cloud or on a local drive, providing flexibility in data management. For those looking into beginner JavaScript open-source projects, Draw.io serves as a practical example of how to build and contribute to open-source tools with JavaScript. If you’re considering diving into such projects, working with experienced JavaScript programmers can greatly enhance your ability to develop and maintain similar tools. Additionally, Draw.io boasts compatibility with nearly all contemporary web browsers, ensuring a seamless user experience across various platforms.
Here are the key features and aspects of Draw.io:
- Open Source. Draw.io is an open-source project, meaning it is freely available for use, and its source code is openly accessible. This makes it highly customizable and adaptable to specific needs.
- Wide Range of Diagram Types. Draw.io supports the creation of various diagrams, including flowcharts, UML diagrams, network diagrams, org charts, mind maps, entity-relationship diagrams, and more. It is a versatile tool suitable for various use cases.
- Customizable Styles and Themes. Users can customize the look of their diagrams by changing colors, fonts, and styles. Draw.io also provides themes and templates to speed up the diagram creation process.
- Auto-Layout and Alignment. The tool offers automatic layout and alignment features, making it easy to keep your diagrams organized and aesthetically pleasing.
- Diagram Versioning. Draw.io supports version control for your diagrams, allowing you to track changes and revert to preceding versions.
Draw.io is a versatile, open-source diagramming tool that offers various features for creating, sharing, and collaborating on diagrams.
Prisma.io
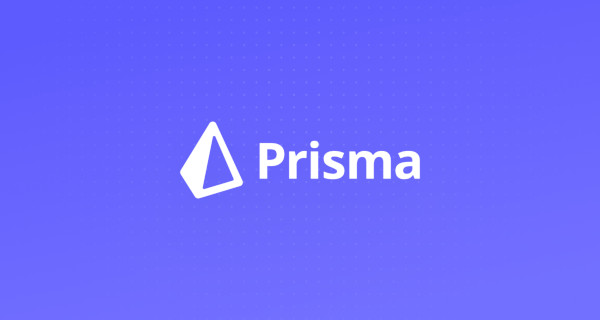
Prisma is a server-side library that helps programmers read and write data to the database intuitively, efficiently, and safely. It offers a set of tools and libraries to streamline database operations, making it easier to work with databases in a type-safe and efficient manner. Prisma.io uses Git for version control to manage changes and collaborate on its development, which is essential for maintaining the account of its ongoing updates and contributions.
Here are the key features and aspects of Prisma:
- Type-Safe Database Access. Prisma provides a strongly typed and auto-generated query API, ensuring that your database queries are type-safe and free from runtime errors.
- Database Agnostic. Prisma supports various relational databases, including PostgreSQL, MySQL, SQLite, and SQL Server. It abstracts the database-specific details, making it easier to switch between different database systems.
- Automatic Schema Migration. Prisma offers a migration tool that helps you manage changes to your database schema. You can create and apply migrations to maintain your DB schema in sync with your application’s code.
- Data Modeling. Prisma lets you define your data model using a simple and intuitive DSL (Domain-Specific Language). This model is then used to generate database tables and the corresponding CRUD operations.
- Query Builder. Prisma provides a query builder that allows you to construct complex database queries using a fluent and chainable API. This feature is particularly useful for filtering, sorting, and aggregating data.
- Real-time Data with Prisma Client Realtime. Prisma supports real-time data synchronization through Prisma Client Realtime. It allows you to subscribe to database changes and receive updates in real-time, making it suitable for building reactive applications.
- Integration with Popular Frameworks. Prisma can be easily integrated with popular backend frameworks such as Express, NestJS, and more, simplifying the complex web development of full-stack applications.
The Prisma schema is intuitive and lets developers declare their database tables in a human-readable way, making the data modeling experience a delight. Developers define their models by hand or introspect them from an existing database. As one of the prominent JavaScript open-source projects on Github, Prisma simplifies database management and is a valuable addition to any JavaScript developer’s toolkit.
Polymer
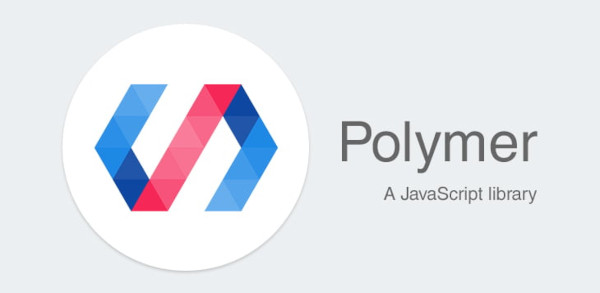
Polymer, an open-source JavaScript library, is designed for constructing applications with “Web Components.” These components offer developers the concept of reusable widgets or web blocks, fostering seamless interoperability among individual web elements. This approach has been harnessed in developing intricate projects, including notable examples such as YouTube and Google Earth.
Here are the key features and aspects of Polymer:
- Web Components. Polymer is centered around the use of web units, a set of web platform APIs that allow developers to create reusable, encapsulated, and composable UI elements. These components include Custom Elements, Shadow DOM, HTML Templates, and HTML Imports.
- Reusability and Encapsulation. Polymer promotes the creation of reusable components that is simple to share and use across different projects. These components are encapsulated, meaning their internal structure and styling are hidden outside, reducing the risk of style and functionality conflicts.
- Declarative Syntax. Polymer uses a declarative syntax to define web components. Component behavior is defined in a way that is similar to writing HTML, making it more intuitive and approachable for developers.
- Lifecycle Callbacks. Web components in Polymer have lifecycle callbacks such as created, attached, and detached, which allow developers to manage component initialization and cleanup. This makes it easier to work with components predictably.
- Events and Gestures. Polymer simplifies event handling and provides a rich set of pre-defined gestures and event listeners for common interactions, making creating interactive and responsive components easier.
- Templating. Polymer uses HTML Templates to define the structure of components. This makes it straightforward to create reusable templates that can be instantiated multiple times with different data.
- Polyfills. Polymer includes polyfills to enable web component features in browsers that do not natively support them. This ensures that web components and Polymer applications work consistently across different browsers.
- Tooling. Polymer offers tools and libraries, including the Polymer CLI, for project scaffolding, development, testing, and optimization. These tools streamline the development process and improve the developer experience.
Polymer is a library that focuses on the use of web components to simplify web development. It promotes reusability, encapsulation, and a declarative syntax for creating web components, making it a valuable choice for developers who want to build modular and maintainable web applications.
MaybeWorks — reliable IT staff augmentation provider
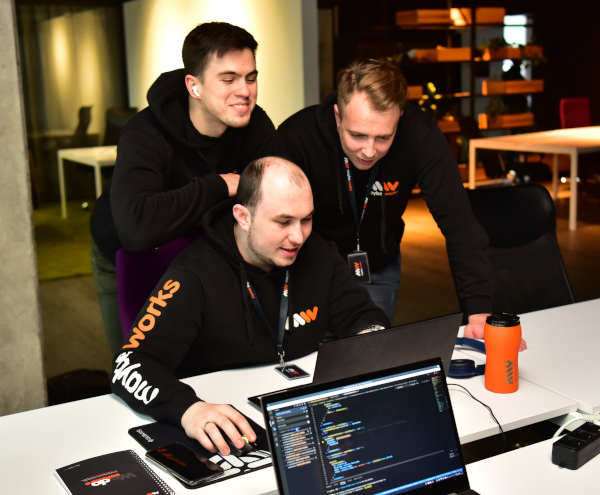
MaybeWorks is a highly reputable IT staff augmentation provider known for its outstanding team of top-notch MERN developers with extensive expertise in various technologies, including Nest, Date-fns, Socket.IO, Axios, and ESlint. Our skilled team of experts has earned a reputation for augmenting development teams and significantly contributing to the success of a wide range of projects. We assisted in developing SaaS, web apps, mobile apps, ERP (Enterprise Resource Planning), CRM (Customer Relationship Management), and more.
Feel free to contact us right now if you need reliable augmented developers for your business.