Understanding React Architecture
React architecture is the way you organize and structure a React application. Think of it as laying the foundation for your app—it sets the stage for everything else.
At its heart, React is built around components, which are like building blocks for your UI. A solid architecture ensures these blocks fit together perfectly, making your app clean, predictable, and easy to work with.
The Importance of Architecture in React Applications
Good architecture isn’t just about writing neat code—it’s about making your app easier to grow, work on, and fix. Here’s why it matters:
- Scalability: As your app grows, a clear structure keeps things from getting messy or hard to handle.
- Teamwork: A well-organized codebase makes it easier for multiple developers to work together without stepping on each other’s toes.
- Debugging: When your app is neatly structured, finding and fixing bugs is way less frustrating.
Benefits of a Well-Structured React App
- Easy Maintenance: Need to add a feature or fix something? You’ll know exactly where to look.
- Reusability: Create components you can use in multiple places, saving time and effort.
- Better Performance: With techniques like lazy loading or memoization, a well-structured React app naturally performs better.
- Future-Proofing: Your app will be ready to handle updates and new features without breaking a sweat.
When your React project is organized by features or domains, you’ll spend less time hunting for files and more time focusing on web development. It’s a simple approach that can make a big difference.
Core Concepts of React
Components and JSX
At the heart of any React app architecture are components, the building blocks of your application, allowing you to break down the UI into smaller, reusable pieces. React uses JSX (JavaScript XML), a syntax that looks like HTML but is written in JavaScript, to define these components. This combination of structure and logic makes React both powerful and easy to work with. These advantages of React—such as component reusability and JSX syntax—make it an ideal choice for building modern, dynamic applications.
Here’s a simple example of a functional component using JSX:
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
React Elements and the Virtual DOM
React elements are the smallest building blocks of React apps representing the structure you want to display on the screen. Behind the scenes, the Virtual DOM (a lightweight representation of the actual DOM) ensures efficient updates.
When you update your user interface, React compares the new Virtual DOM with the previous one, identifies changes, and updates only the affected parts of the actual DOM. This process, known as reconciliation, boosts performance and is a key part of React performance optimization.
Here’s an example to illustrate the Virtual DOM in action:
const element = <h1>Hello, word!</h1>;
ReactDOM.render(element, document.getElementById('root'));
State and Props in React
State and props are how React components communicate and manage data. Props are used to pass data from a parent component to a child component, while state is managed within a component and can change over time. Together, they form the foundation of dynamic UIs.
Example of state and props:
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Common React Architecture Patterns
Functional Components vs. Class Components
Functional components and class components both serve the same purpose—defining your UI and its behavior—but they go about it differently.
Functional components are essentially JavaScript functions that return JSX. They’re simple, clean, and easy to understand. And thanks to hooks, which were introduced in React 16.8, functional components can handle state and side effects just as well as class components.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Class components, on the other hand, were the original way to handle state and lifecycle methods in React. They use ES6 classes and include methods like componentDidMount and shouldComponentUpdate to manage the app’s behavior over time.
import React, { useState } from 'react';
function Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
While class components get the job done, they’re a bit bulkier and harder to follow compared to functional components.
Container and Presentational Components
Presentational components focus purely on how things look—they handle the UI and don’t worry about data or logic. Container components manage the logic and data flow, leaving the UI details to their presentational counterparts.
Higher-Order Components (HOC)
Higher-Order Components (HOCs) are a powerful pattern in ReactJS architecture for reusing component logic. Think of an HOC as a function that takes a component and returns a new component with added functionality. For example, you could use an HOC to inject data from an API into multiple components. The beauty of HOCs is that they let you keep your components clean while sharing common logic across them.
Render Props Pattern
This pattern is useful when you need to share logic, like handling user input or fetching data, without duplicating code. Instead of hardcoding UI, you pass a function as a prop to a component, which then uses that function to render its UI.
Compound Components
Working together as a unit but each handling a specific part of the UI, they make it easier to share state and logic between components without prop-drilling.
It’s great when you need fine-grained control over how parts of the UI behave together, like in form controls or complex navigation components. For example, during Angular to React.js migration, compound components can simplify your UI structure, making it more flexible and easier to manage.
Controlled and Uncontrolled Components
Controlled components have their state managed by React. For instance, form inputs are controlled when React handles their value and updates. Uncontrolled components handle their own state internally, often using the DOM directly.
While uncontrolled components are simpler to set up, controlled ones offer more flexibility and are generally preferred for more complex forms or interactions.
Organizing Your React Project Structure
Directory Layout Best Practices
A well-structured directory layout is the backbone of any React project architecture. Keeping your files organized makes your code easier to navigate, maintain, and scale as your app grows.
A clean architecture layout saves time for you and your team, ensuring everyone knows where to find what they need. For example:
src/
components/
assets/
utils/
pages/
Avoid cramming everything into one folder or creating unnecessary nesting—it’s all about balance.
Grouping by Feature vs. Function
Grouping can shape how manageable your project feels. Grouping by feature keeps all related files (components, styles, tests) in one place, making it easier to work on specific parts of the app. Grouping by function, like having a global folder for components or styles, can work for smaller projects but often gets messy as the app grows.
In offshore React.js development, grouping by feature is usually more effective. It keeps things clear and organized for teams collaborating across time zones.
Incorporating Custom Components in Folders
Create a dedicated folder, such as src/components, and group related components into subfolders. For example:
src/
components/
Button/
Button.js
Button.css
Modal/
Modal.js
Modal.css
This structure makes it easier to locate, update, or reuse components without unnecessary digging. Naming folders after components also simplifies navigation in larger projects.
Utilizing Absolute Imports
Remove the hassle of lengthy relative paths: instead of navigating folder hierarchies like ../../components/Button, you can reference components directly, such as components/Button. If you’re working on a large project, absolute imports make it much easier to move files around without breaking your imports—particularly useful during refactoring or when collaborating on complex applications.
State Management Strategies
Local State Management with Hooks
Managing local state with React’s built-in hooks, like useState and useReducer, is an intuitive and lightweight approach for handling state within React components. These behavior hooks shine for managing simple state requirements, such as form inputs or toggles.
Global State with Context API
Ideal for managing global state that needs to be shared across multiple components, like user authentication or theme settings. It’s best suited for smaller apps or lightweight global state. For larger projects, its overuse can lead to performance bottlenecks, making it wise to consider alternatives.
Using State Management Libraries (Redux, MobX)
When your app’s state becomes complex, libraries like Redux or MobX are invaluable. Both tools bring scalability and clarity to React application architecture patterns: Redux offers a predictable state container with a strict structure, ideal for larger apps requiring robust debugging tools. MobX provides a more flexible, reactive approach, often favored for simpler, dynamic use cases.
Separating Business Logic from UI Components
Move logic-heavy tasks, like API calls or state transformations, to dedicated helper functions or custom hooks. This keeps your UI components clean and makes your app easier to test and maintain, especially in larger projects.
Creating Reusable Components
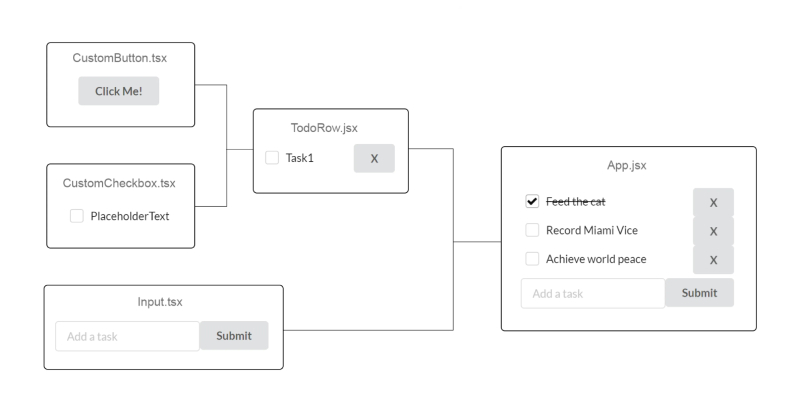
Designing Custom Components
Create reusable components in React’s component-based architecture to encapsulate specific component behavior, like buttons, cards, or modals. Keep them modular and focused, so they can be easily integrated into various parts of your React codebase.
Implementing Custom Hooks
Custom hooks are powerful when sharing business logic across multiple components, simplifying your application structure. For example, a custom hook for fetching data from a services API can standardize how components handle loading states and errors, keeping your frontend development clean and efficient.
Sharing Logic Across Components
React simplifies sharing data and logic with context, props, or higher-order components, but custom hooks excel at centralizing complex data. This reduces redundancy and keeps frontend components focused on rendering.
Working with Side Effects
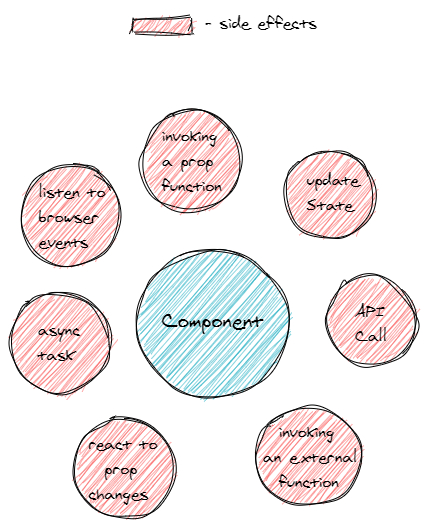
Understanding the useEffect Hook
The useEffect hook handles side effects like data fetching, subscriptions, or DOM updates. It runs after the render phase, and your app stays in sync with external changes. To avoid performance issues, always clean up subscriptions or event listeners in the effect’s cleanup function.
Managing Data Fetching and Subscriptions
Careful use of useEffect with dependencies prevents memory leaks and unnecessary re-renders. For complex apps, custom hooks and libraries like Axios streamline React micro frontend best practices for clean side effect management.
Handling Asynchronous Operations
Async tasks like API calls benefit from custom hooks for managing loading states and errors. Using async/await boosts readability, contributing to a solid architecture of ReactJS that supports scalable, efficient apps.
Performance Optimization Techniques
Code Splitting and Lazy Loading
With React.lazy() and Suspense, you can load only the necessary parts of your app, reducing the size of your initial JavaScript bundle. If you’re exploring alternatives to React, code splitting can boost performance in other frameworks too.
Memoization with React.memo and useMemo
Use React.memo() for functional components and useMemo() for values or calculations that don’t need to re-run on every render. These hooks help avoid unnecessary re-renders, improving app performance, especially in larger apps with complex components.
Optimizing Rendering with shouldComponentUpdate
shouldComponentUpdate is a lifecycle method in class components that prevents unnecessary renders by returning false when the component doesn’t need to update. In functional components, React.memo() serves a similar purpose in front end applications, optimizing rendering by comparing props.
Styling Patterns in React
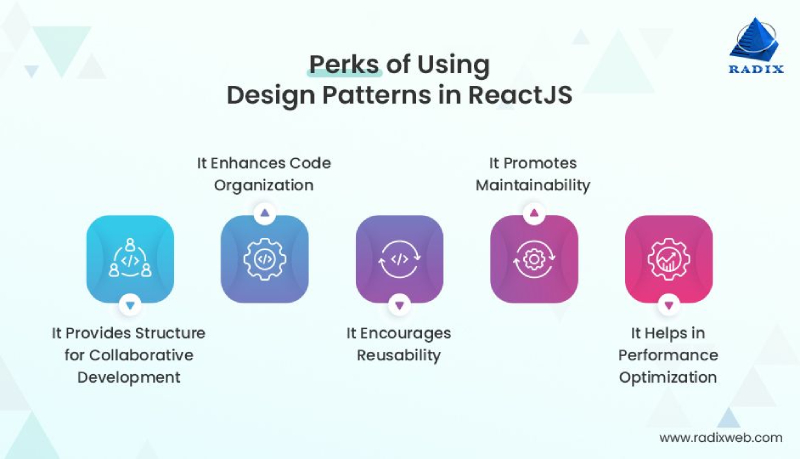
CSS-in-JS Solutions
CSS-in-JS libraries like styled-components or emotion let you write CSS right in your JavaScript code. This approach keeps styles tied to components, which is especially handy in larger React applications where managing styles can get tricky. If you’re looking to streamline your project, consider React.js developers for hire to help integrate such solutions into your workflow.
Using Styled Components
Styled Components align with best practices in React JS application architecture: by combining the logic and styling into one, they make things cleaner and more manageable—plus, they let you create dynamic, state-based styles that respond to your component’s needs.
CSS Modules and Scoped Styling
CSS Modules take the guesswork out of styling by automatically generating unique class names for each component. This keeps styles isolated, avoiding the dreaded global style conflicts, and fits perfectly with a component-based architecture in your web applications.
Traditional CSS and BEM Methodology
While newer techniques are gaining popularity, traditional CSS and the BEM (Block, Element, Modifier) methodology still have their place. BEM provides a clear structure, helping you maintain best practices as your React web application grows.
Testing and Debugging React Applications
Unit Testing with Jest
Jest simplifies unit testing by providing built-in mocking, assertions, and coverage reporting, ensuring your front end code works as expected. It’s ideal for testing isolated React components to ensure they behave correctly and catch issues early.
Component Testing with React Testing Library
React Testing Library tests components from the user’s perspective, ensuring they’re accessible and function as expected. It focuses on simulating real-world interactions, making components more reliable and user-friendly and ensuring smooth data flow.
End-to-End Testing with Cypress
Cypress enables end-to-end testing by simulating user interactions and checking overall app functionality. It’s a great solution for catching integration bugs in the architecture of React application and ensuring everything works seamlessly together.
Debugging Techniques and Tools
Tools like React Developer Tools and Chrome DevTools help inspect the component tree, state, and props. Proper error handling and error boundaries improve debugging and ensure bugs are caught early.
Advanced React Patterns
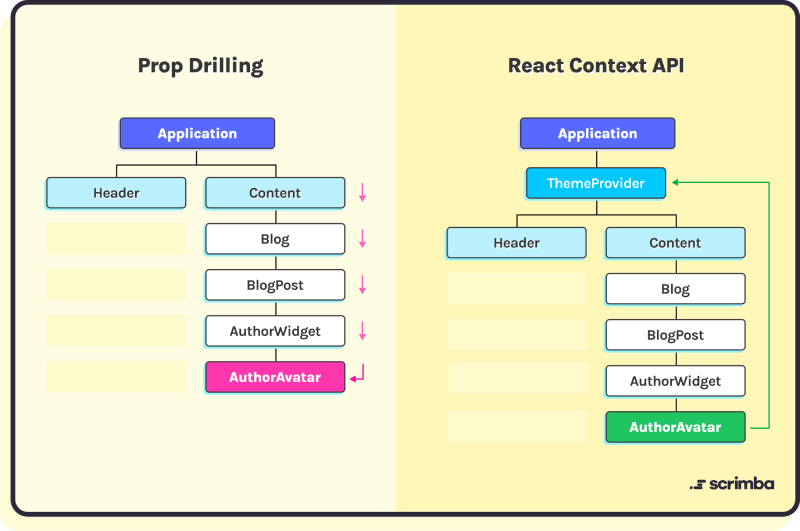
Context API Patterns
This tool allows you to share values like authentication, themes, or settings across your entire web application without prop drilling. Perfect for passing data through many layers of your React component tree. Use it when you need global state management without relying on external libraries like Redux.
Render Props vs. Hooks
Both share logic between components but differ in approach: render props pass a function as a prop to children components, and hooks encapsulate reusable logic and state management inside function components. While render props remain useful, hooks offer a cleaner way to manage state and side effects, fitting well with architecture CSR in web development.
Prop Drilling and How to Avoid It
Prop drilling happens when you pass props through many layers of components to get them to the component that needs them. This can quickly make your app development slower and harder. Instead, use React’s Context API or state management libraries like Redux to keep your code more maintainable and readable.
If you’re looking to scale your team, check out our article on the cost to hire React developers.
Implementing Error Boundaries
Error boundaries are part of React architecture patterns designed to catch errors in components. They prevent the entire app from crashing by gracefully handling exceptions within specific components. Wrapping your src components in error boundaries ensures that an error in one part of the app won’t affect the rest—a top practice for maintaining app stability.
Best Practices for Scalable React Apps
If you want your React app to grow smoothly, sticking to best architecture practices is the way to go.
Code Organization and Maintainability
Organizing your codebase is key when building scalable React apps. Keeping folder structure clean and grouping related components together is all about setting up a frontend architecture that allows for easier updates and changes down the line. If you’re thinking about alternatives, our React.js vs. Angular guide might help you choose the best framework based on your project needs.
Documentation and Commenting Standards
Documentation and commenting standards may not seem thrilling, but they’re invaluable for React web development and other processes like a React to NextJS migration. Clear comments on functions and components make understanding and collaboration easier, preventing costly errors later on.
Avoiding Common Pitfalls
Steering clear of missteps like prop drilling or unnecessary state updates is something you’ll want to watch out for. Stick to best practices for state management and re-renders, and you’ll keep your app running without making things more complicated than they need to be.
Need a hand with optimizing your React development? The team at MaybeWorks has got you covered. Let’s chat and make your project a success!
Utilizing the Utils Directory
Using a utils directory to store reusable functions is a small habit that makes a big impact. It keeps your code DRY (Don’t Repeat Yourself) and ensures your design components stay clean and focused on their job. This is just one of the React architecture best practices to keep things organized and easy to maintain.
Accessibility in React Applications
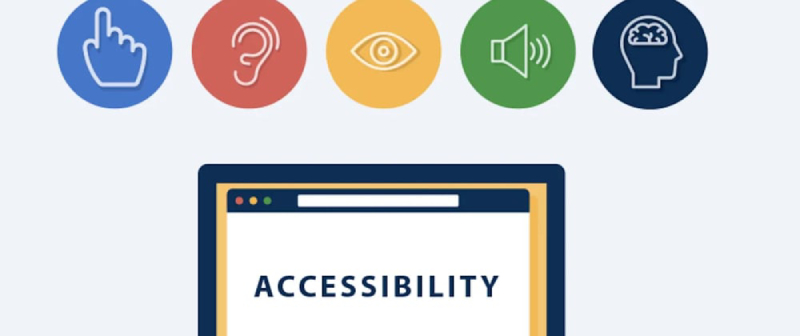
Importance of Web Accessibility
Creating a straightforward single page web application or a sophisticated MPA? Accessibility should be a priority, ensuring your app works for everyone—in bright sunlight, low light, or noisy settings. It’s a key part of React web application architecture, making apps more inclusive and user-friendly in any situation.
Implementing ARIA Roles and Attributes
Adding ARIA roles and attributes helps improve navigation and interaction across your app. These specifics make component details more understandable for screen readers and other assistive tools, ensuring clarity for all users, no matter how they access your app.
Tools for Testing Accessibility
Tools like Axe, Lighthouse, and WAVE are great for spotting accessibility issues early on. Using them ensures your assets components follow a solid design pattern, promoting user-friendly and accessible design from the ground up.
Deploying React Applications
Preparing for Production Build
Getting your React app production-ready involves optimizing assets and code. Tools like Webpack or Vite help you bundle your app efficiently, ensuring faster load times and better performance, even in complex setups like micro frontend architecture.
Continuous Integration and Deployment (CI/CD)
Setting up CI/CD pipelines simplifies deployment, making it easy to roll out updates without stress. It’s especially handy for apps with micro frontend components, letting you make changes without impacting the rest of the app.
Monitoring and Performance Tracking
Keeping an eye on your app’s health after deployment is a must. Tools like Sentry and New Relic make it easy to monitor performance and tackle issues, whether they’re on the client or server side, ensuring everything runs smoothly for your users.
Conclusion
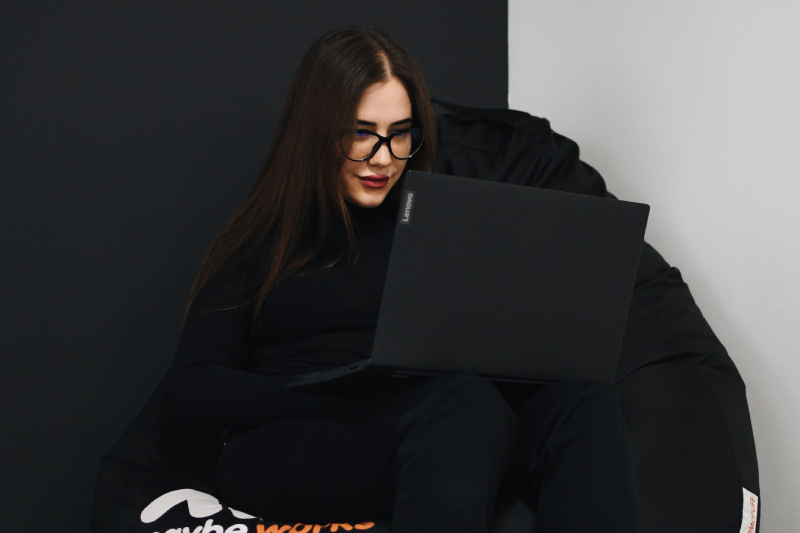
Building scalable and efficient React apps means paying attention to all the important details—from accessibility and design patterns to smooth deployment. With the right React framework architecture and software, you can ensure your app is high-performing, inclusive, and ready for anything.
Ready to take your app to the next level? Get in touch with MaybeWorks and let’s make it happen!