Overview: React Micro Frontend Overview
React micro frontends are all about breaking down big, monolithic frontends into smaller, manageable pieces. Each piece (or micro app) can be built, tested, and deployed independently, making it easier to scale and maintain. If you’re considering an Angular JS to React migration, this approach can help you avoid the pain of massive rewrites while giving you more flexibility and speed in your development process. It’s a game-changer for teams working on complex web applications.
Benefits of Using React Micro Frontend
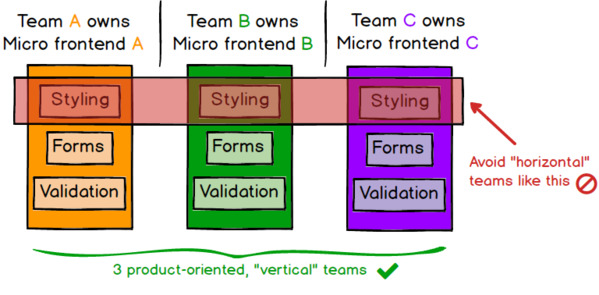
Scalability and Flexibility
When you’re dealing with complex frontend applications, adopting micro frontend React gives you the flexibility to scale individual pieces of your app independently. This approach improves your team’s ability to manage large projects, simplifies deployment of each module, and works even better with React development best practices.
Faster Development Cycles
By splitting your app into independent microservices, teams can work in parallel without stepping on each other’s toes. Whether you’re working with an in-house team or considering offshore React development, micro frontends speed up your release cycles by isolating feature development and reducing the risk of breaking other parts of the app.
Easy Integration with Other Tools and Frameworks
Thanks to Module Federation, integrating various frameworks or tools becomes more manageable. You can combine different technologies like React and Angular under the same roof, making it a great fit for hybrid tech stacks. Plus, it’s seamless when moving from monolithic frontend setups to more modular systems.
To get a better understanding of the financial impact of adopting such solutions, check out our article on the cost of hiring a remote React.js developer for your project.
Tradeoffs in React Micro Frontend Development
While React micro frontends offer a lot of benefits, they come with a few challenges too.
More Moving Parts
Sure, MFE in React gives you flexibility, but now you’re juggling multiple codebases and microservices. More pieces can mean more headaches, especially when trying to keep everything in sync.
Performance Might Suffer
Each micro frontend application brings its own resources to the table, which can slow things down. The trick? Optimize your web applications to keep things running smoothly without bloating your app.
Team Coordination Gets Tricky
More teams, more freedom—but also more chances for chaos. Keeping React DOM structures and shared dependencies consistent across the board can take extra effort when working with MFE architecture React.
Understanding Module Federation
Module Federation is a powerful feature that enables ReactJS micro frontend applications to share code seamlessly across different micro frontends. Implement it, and you will avoid redundancy and keep your application’s bundle size down, all while enhancing user experience. If you’re looking to implement on-the-fly functionalities, integrating React WebSockets can further enhance responsiveness and interactivity in micro frontend setups.
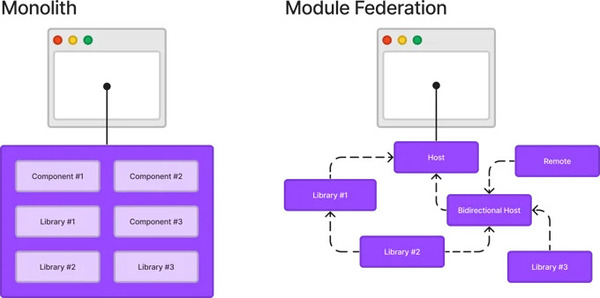
Instead of loading everything separately, you can efficiently share libraries and components, making your app lighter and faster. Imagine a remote app that can pull in the latest versions of shared components without hassle—this is the beauty of Module Federation in action! Don’t forget to apply React performance optimization techniques—the combination of two ensures your app runs smoothly and offers a great experience for users.
React’s Role in Microfrontend Architecture
React is a real game-changer in MFE thanks to its component-based design. For what is React JS? This library allows developers to build independent, reusable components that fit together like puzzle pieces. This modular approach not only makes it easier to scale applications but also helps keep things organized as the project grows.
When you’re using React for micro frontends, it’s a good idea to adopt a micro UI architecture of React application to ensure consistency across different teams. Techniques like lazy loading and code splitting can boost performance and help manage shared libraries better.
For example, when you import React in various micro frontends, it keeps each component lightweight and manageable. Keeping a strong microservices architecture allows for easy integration of all components without losing performance. This approach also aligns with SEO in React.js strategies, ensuring that your app remains optimized for search engines while maintaining a modular structure.
How to Build React Micro Frontend Architecture
Building a React micro frontend architecture is pretty straightforward. Here’s how to create React app micro frontend, focusing on key concepts:
- Set Up Your Main Application:
- Start by creating your main app using Create React App. This will serve as the shell for your React micro frontends.
- Organize your project structure clearly, with folders for components, services, and shared utilities. For layout, use
div className
to structure your components effectively.
- Create Micro Frontend Projects:
- For each feature or section (e.g., user profile, admin panel), create separate frontend applications using micro architecture. Create React App would be great for consistency here.
- Each micro frontend should be self-contained, including its own routing and state management.
- Implement Module Federation:
- In your main application, configure Webpack 5’s Module Federation plugin. This allows you to dynamically load your micro frontends at runtime.
- Define shared dependencies (like React and ReactDOM) in your Webpack configuration to avoid duplication.
- Handle Routing:
- Use React Router in your main application to manage navigation between micro frontends.
- Each micro frontend can define its routes, and the main app can load the appropriate micro frontend based on the current path.
- Manage Shared State:
- To maintain a smooth user experience, use a state management solution.
- Use React Context for lightweight state sharing or Redux for more complex state management across micro frontends.
- Communication Between Micro Frontends:
- Use a custom event system or a pub/sub pattern for communication between micro frontends, allowing them to react to changes or updates from each other.
- Testing and Quality Assurance:
- Write unit tests for each micro frontend independently. Take Jest or React Testing Library for component testing.
- Perform integration tests to ensure that micro frontends work together as expected.
- Deployment:
- Choose a deployment strategy that allows each micro frontend to be deployed independently. Tools like Docker or cloud platforms like AWS, Vercel, or Netlify work well for this.
- Automate your deployment process using CI/CD pipelines to streamline updates and ensure consistent releases.
By following these steps, you can create a solid React micro frontend architecture that scales well and keeps your code clean.
Best Practices for React Micro Frontends
This tutorial will guide you through achieving optimal results, giving you insights that lead to the best micro frontend React example for your projects.
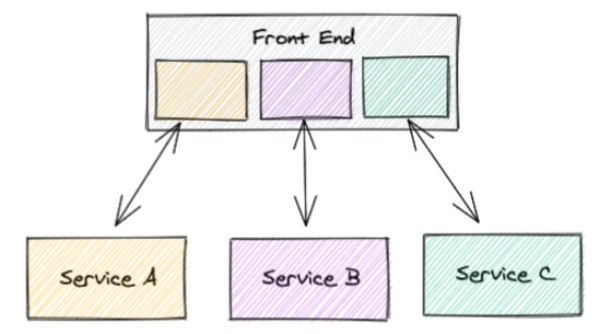
- Consistent Communication Protocols:
- Establish clear communication between micro frontends. Using a well-defined API or event bus can simplify data sharing and updates. You might consider using event emitters to facilitate interaction between components.
- Maintain Modular Design:
- Keep each micro frontend focused on a specific feature or functionality. This modular approach not only improves maintainability but also makes it easier to scale. The advantages of using React JS shine here, as its component-based structure enables smooth scalability and easier maintenance.
- Use Version Control:
- Version control allows teams to deploy independently without affecting the overall application. Ensure your repository structure supports individual micro frontend projects.
- Local Development Environment:
- When developing locally, run your applications using
http://localhost:3000
for easy access and testing. This setup allows you to see changes in real-time as you build.
- When developing locally, run your applications using
- Performance Optimization:
- Optimize each with techniques like lazy loading and code splitting. They help reduce initial load times and improve user experience.
- Testing Is Key:
- Implement automated testing for each micro frontend. Use unit tests to verify functionality and integration tests to ensure flawless interaction with other micro frontends.
- Continuous Integration/Continuous Deployment (CI/CD):
- Automate your deployment process using CI/CD pipelines. This ensures that updates are deployed consistently and efficiently across all micro frontends.
- Consider Framework Comparisons:
- If you’re weighing options, consider the differences between frameworks. Understanding React vs Angular can guide your choice based on your project’s specific needs.
How MaybeWorks Can Help?
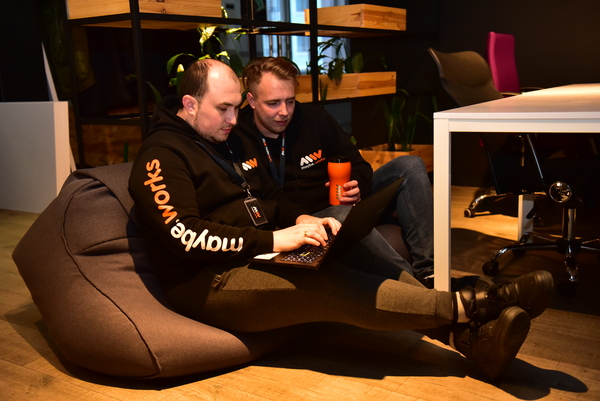
At MaybeWorks, we know how to create a micro frontend React that makes your app easier to manage and scale. Here’s how we can support your development process:
At MaybeWorks, we help you break down your monolithic apps into smaller, manageable micro frontends, with clear APIs for smooth integration. We also set up Webpack 5’s Module Federation, so you can load remote micro frontends dynamically, making your app more efficient. To keep things consistent, we manage shared state with tools like Redux or Recoil. For better performance, we use lazy loading and code splitting to speed up load times. And to make sure everything runs smoothly, we establish automated testing and CI/CD pipelines for reliable deployments.
Ready to level up your application? Learn how to hire React.js developers who can help you build a scalable micro frontend architecture. Reach out to us today!
Conclusion
Adopting a micro frontend architecture in your React projects can significantly enhance scalability and team collaboration while improving user experience. By breaking down large applications into smaller, manageable parts, you’ll streamline your development process. Whether you’re starting fresh or optimizing an existing setup, embracing this approach will set you up for success in today’s dynamic web landscape.
If you have questions or need assistance, feel free to contact us!
FAQ
-
How does implementing micro frontends with React enhance application scalability and team productivity?
A React micro frontend architecture splits the app into smaller modules, making it easier to scale. Teams can work independently on different features, speeding up development and reducing complexity.
-
How can you manage shared state and data synchronization across different React micro frontend modules?
Shared state is handled through services or libraries. Using Module Federation in frontend microservices React makes it easy for different modules to share data, keeping everything consistent across the app.
-
What strategies do you use to ensure consistent styling and theming across independent micro frontends in React?
A shared design system or micro frontend framework in React ensures that all micro apps follow the same styling, keeping the user experience uniform across the application.
-
How do you handle routing and navigation between different micro frontends in a React application?
We use a central router in the main application to manage cross-app navigation, while each microfrontend React handles its own internal routing.
-
Can you explain how to optimize performance when integrating multiple React micro frontends?
By using Module Federation plugin and lazy loading, each microfrontend only loads necessary resources, speeding up the overall React application and enhancing performance.
-
What are the security considerations when deploying React micro frontends, and how do you address them?
Each microservice should be sandboxed, with secure API communication and proper authentication at the micro-app level to keep data safe.
-
How do you approach testing and quality assurance in a React micro frontend architecture?
All micro components in React are tested individually with unit tests, while integration tests ensure micro apps work well together within the application setup.
-
What tools or frameworks do you recommend for building and deploying React micro frontends?
Webpack 5 with Module Federation is great for integration. Starting with Create React App for individual micro apps and using Docker for deployment is a solid approach.
-
Can you share an example where you successfully migrated a monolithic React app to a micro frontend architecture?
Absolutely! We’ve helped clients move from big monolithic apps to a React JS micro frontend setup, making everything faster and easier to manage. If you’re interested in seeing our work, feel free to reach out to us!