In this React WebSocket tutorial, we’ll walk you through the basics, share some examples, and show you just how easy it is to get started. Let’s dive in!
Understanding WebSockets
WebSockets are the backbone of real-time apps. They let your app and the server talk to each other continuously without needing to refresh or repeatedly send requests. Unlike traditional HTTP, React WebSocket keep a connection open, so data flows instantly in both directions.
In React, working with WebSockets feels natural, especially when building things like live chats, notifications, or real-time dashboards. With the WebSocket API, you can set up two-way communication and handle real-time updates effortlessly. This is one of the major advantages of React—its ability to manage complex, real-time features with ease while maintaining smooth performance.
Here’s what makes WebSockets tick:
- Persistent connection: The link stays open, so data can move back and forth without interruption.
- Two-way communication: Both the server and client can send messages whenever needed.
- Efficient updates: No constant polling, saving time and resources.
If you’re interested in a deeper dive into using WebSockets with React, check out the full article about the pros and cons of WebSockets connections on our blog.
Now, let’s see how to get this working in your React app with some practical examples.
Integrating WebSockets with React
Why Use WebSockets in React Applications?
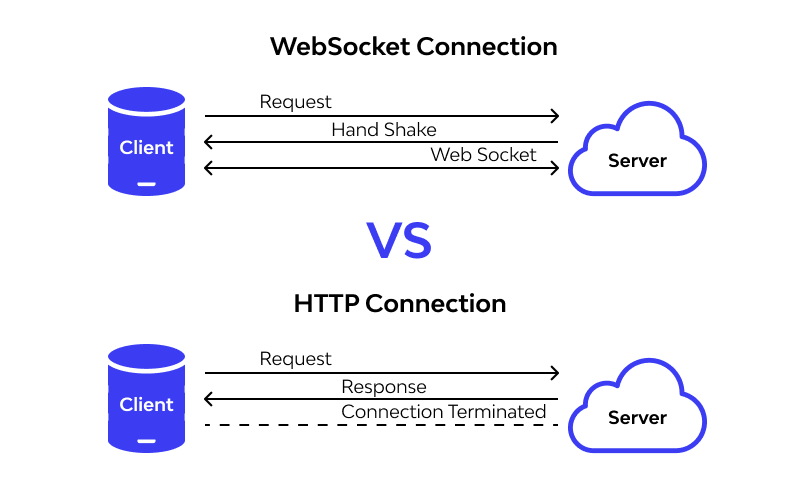
WebSockets are a perfect fit for real-time features in React apps, like live chats, notifications, or collaborative tools. They let your app and server send data instantly without constant requests, which makes everything faster and smoother for users.
When learning how to use WebSockets in React, you’ll find they integrate well with React’s state and lifecycle methods, making it easy to create dynamic, interactive components. WebSockets also enhance user experience by delivering real-time updates seamlessly.
Overview of WebSocket Libraries for React
You don’t have to start from scratch when adding WebSockets to your React app—several libraries make it easier:
- Socket.IO: Great for handling complex real-time features with automatic reconnection and room management—ideal for managing multiple WebSocket connections and real time communication.
- React-use-websocket: A React hook that simplifies connection state management and works well with functional components.
- WebSocket API: Built into browsers, perfect for lightweight, custom setups when you have full control over the WebSocket connection.
- Stomp.js: Ideal if you need support for the STOMP communication protocol, especially when working with structured message to the server logic.
Choosing the Right WebSocket Library
Picking the best WebSocket library for React app depends on what you’re building and how you want to work with it. Not all libraries are created equal, and the right choice makes things easier for you while keeping your app scalable and efficient. Consider factors like ease of integration, support for features like automatic reconnections, and whether the library fits with your app’s architecture.
Here’s what to think about:
- How complex is your app? If it’s a simple project with a few WebSocket interactions, you might not need a feature-packed library. But for something big, like a live chat app with thousands of connected clients, you’ll need something robust.
- How easy is it to use? Some libraries, like react-use-websocket, fit naturally with React and don’t require much setup. Others might take more effort to integrate.
- What features do you need? Do you want automatic reconnections, built-in room management, or support for a specific communication protocol like STOMP?
- Can it handle scale? If your app will have many WebSocket connections at once, make sure the library can keep up without slowing down.
- Is it well-documented? A library with clear documentation and an active community makes troubleshooting and learning much easier.
If you’re still unsure which library suits your project or want to get things done faster, you can hire React.js programmers from MaybeWorks to handle the integration for you.
Setting Up a WebSocket Serve
Implementing a WebSocket Server with Node.js
Setting up a WebSocket server with Node.js is easier than you might think. You can use libraries like ws to manage React WebSocket connection effortlessly. Here’s a quick example:
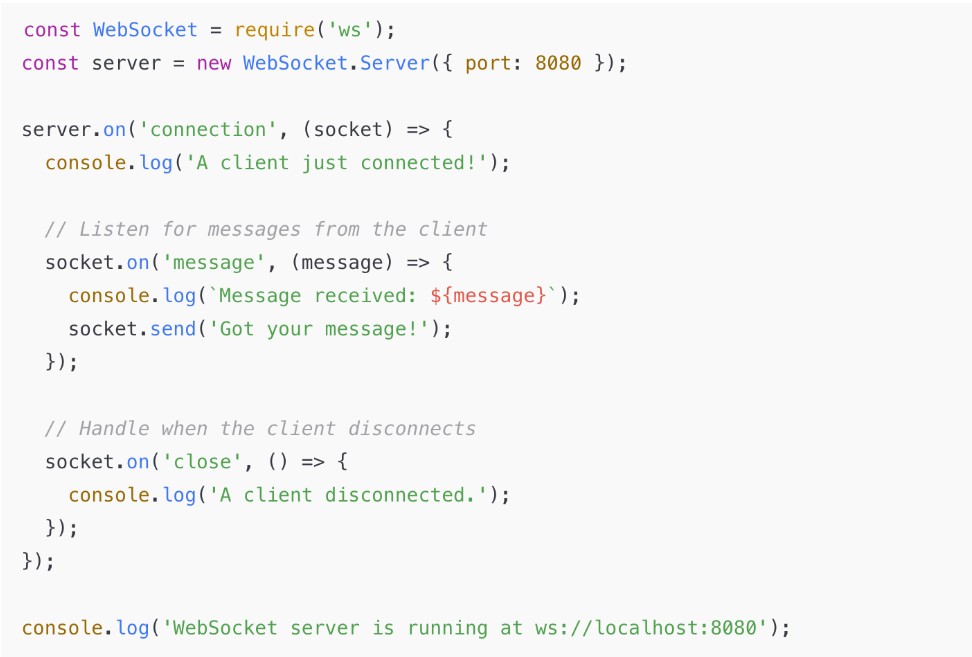
This simple setup lets your server handle real-time communication with clients. It’s flexible enough for a variety of use cases, whether you’re building a chat app or sending live notifications.
Setting Up WebSocket Endpoints
WebSocket endpoints keep your app organized, especially if you’re handling multiple types of communication like chats, notifications, or game updates. You can use URL paths to separate logic for each task:
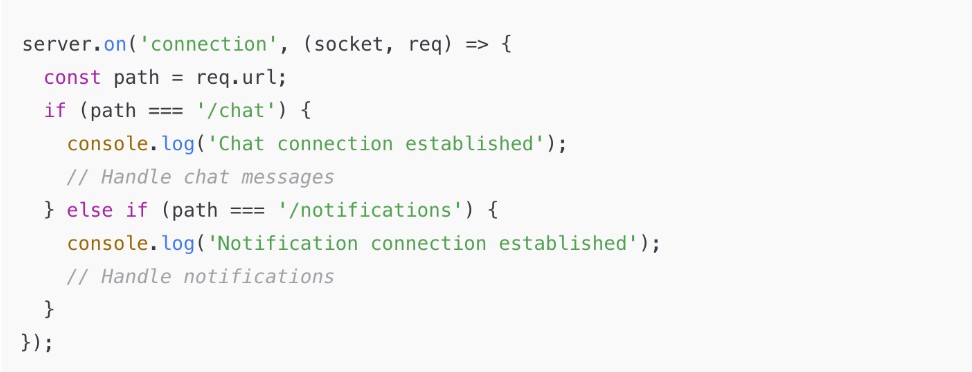
This makes it easy to handle different types of messages without mixing up logic, keeping things clean and scalable.
Handling Client Connections and Messages
When you have multiple connected clients, managing them efficiently is key. Here’s how you can track and handle messages:
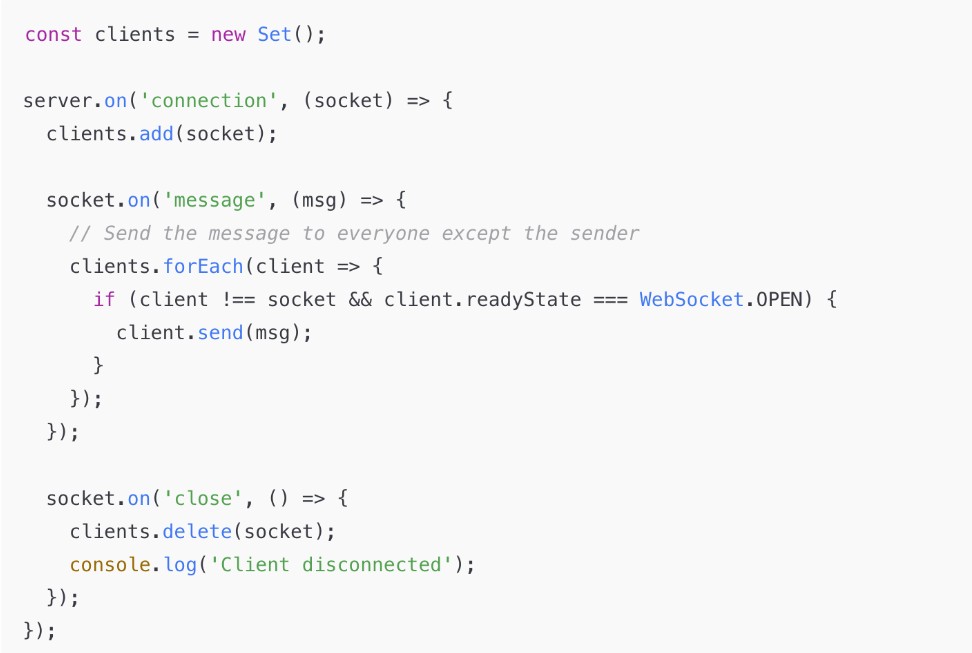
With this setup, you can broadcast updates to multiple users in real-time—ideal for scenarios like live event streaming, online gaming, or stock market trackers.
Establishing WebSocket Connections in React
Creating a WebSocket Connection in React
To create a WebSocket connection in React, you’ll typically use the native WebSocket API or a custom hook, depending on your needs. Here’s a basic way to set up a connection:
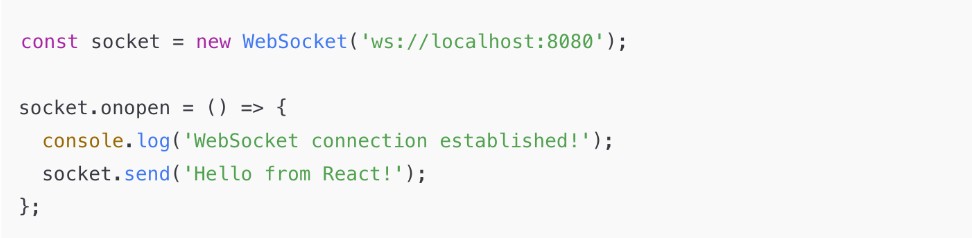
Once the connection is open, you can start sending messages. It’s that simple! The connection is ready for real-time web communication between your React app and the WebSocket server.
Managing the WebSocket Lifecycle
It’s super important to manage the WebSocket React lifecycle, so your app doesn’t run into issues with connection, disconnection, or handling errors. Here’s a quick way to set up lifecycle management using React’s useEffect:
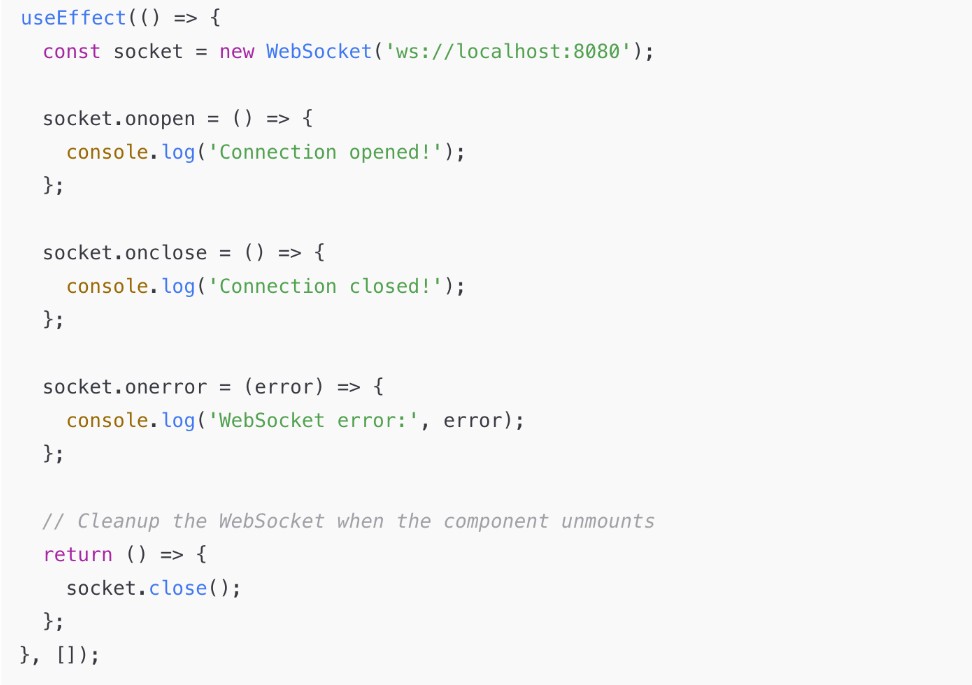
What this does is ensure that when your component is mounted, it opens the WebSocket connection, and when the component is unmounted, it cleans up by closing the connection. This prevents any potential memory leaks or lingering connections.
For more tips on maintaining a lean and efficient app, check out how to improve performance of React application.
Handling Incoming and Outgoing Messages
Now for the fun part: handling bidirectional communication. You’ll want to listen for incoming messages from your ReactJS WebSocket server and also send messages to it. Here’s how to set that up in React:
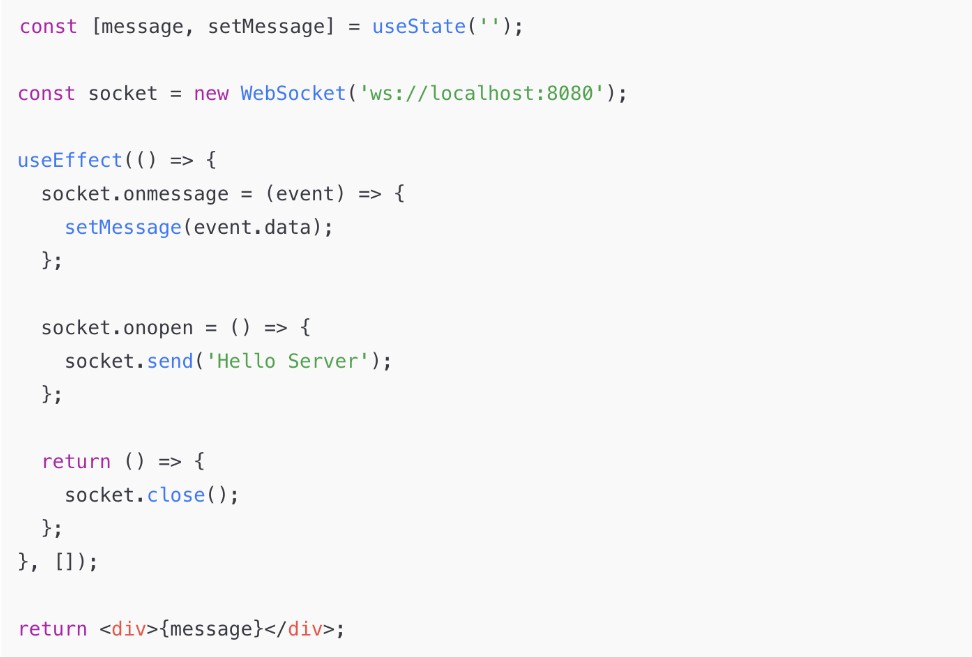
In this example, you’re handling communication between the client and server. When the WebSocket connection is open, the app sends a message to the server. Then, it listens for incoming messages and updates the UI with the response. It’s a simple and effective way to keep your app in sync with real-time data.
If you’re looking to scale your project, consider working with an offshore React development team to get the expertise you need.
Using React Hooks with WebSockets
Implementing useEffect for WebSocket Connections
When working with React and WebSockets, useEffect is a game-changer for managing the WebSocket connection lifecycle. It lets you set up the connection when the component mounts and clean it up when it unmounts, ensuring there are no lingering connections. Here’s how you can do it:
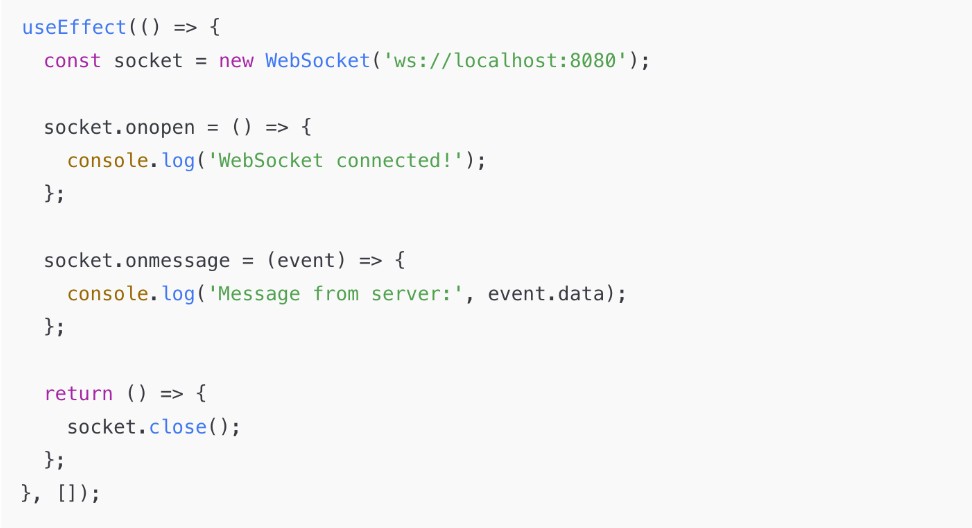
With this setup, useEffect takes care of opening and closing the WebSocket connection for you automatically based on the component lifecycle, keeping things clean and simple. It’s a practical approach when using WebSockets in your app, as it ensures that the connection is properly managed without having to manually handle it on the client side.
Creating Custom Hooks for WebSockets
If you find yourself needing to use WebSockets in multiple places across your app, it might make sense to create a custom hook. A custom hook is a great way to encapsulate WebSocket logic in a reusable and clean way. Here’s a basic example using a React WebSocket hook:
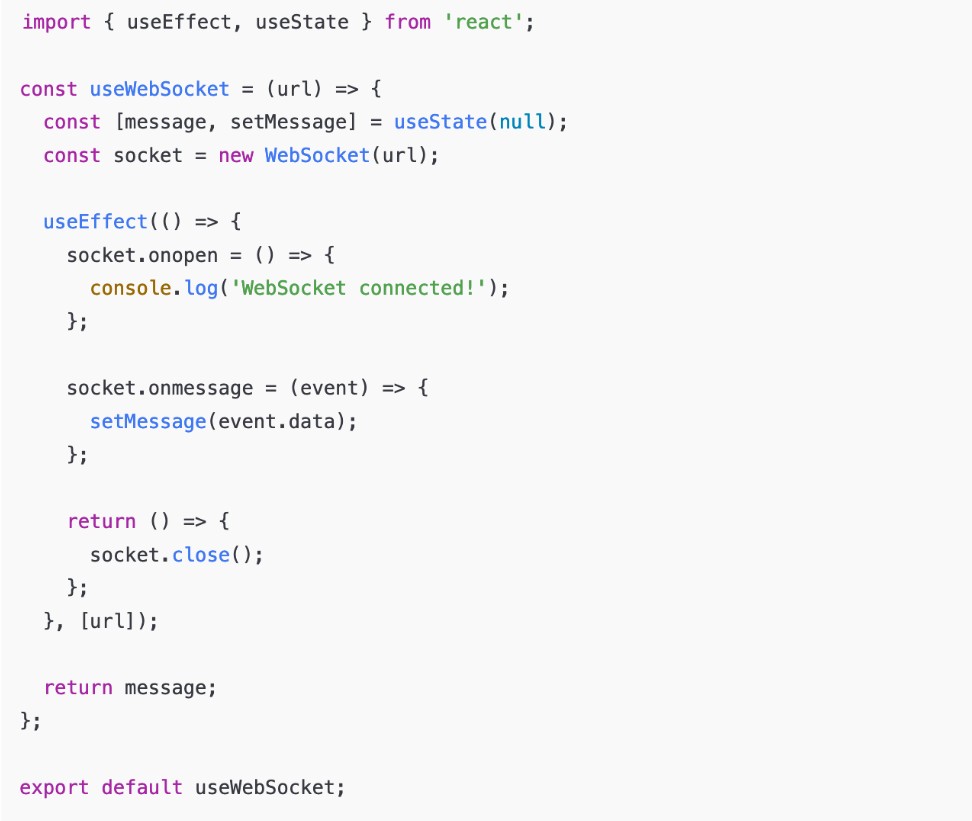
Now you can just call useWebSocket anywhere in your app and pass the WebSocket URL. This keeps your code DRY (Don’t Repeat Yourself) and makes it much easier to manage WebSocket connections across different components—great for managing real-time data across your application.
Leveraging useWebSocket for Simplified Management
If you’re looking to simplify your WebSocket management, you can use the useWebSocket hook, which abstracts the entire connection and message handling for you. There are also third-party libraries, like react-use-websocket, that offer built-in hooks for this purpose. Here’s how you might use it:
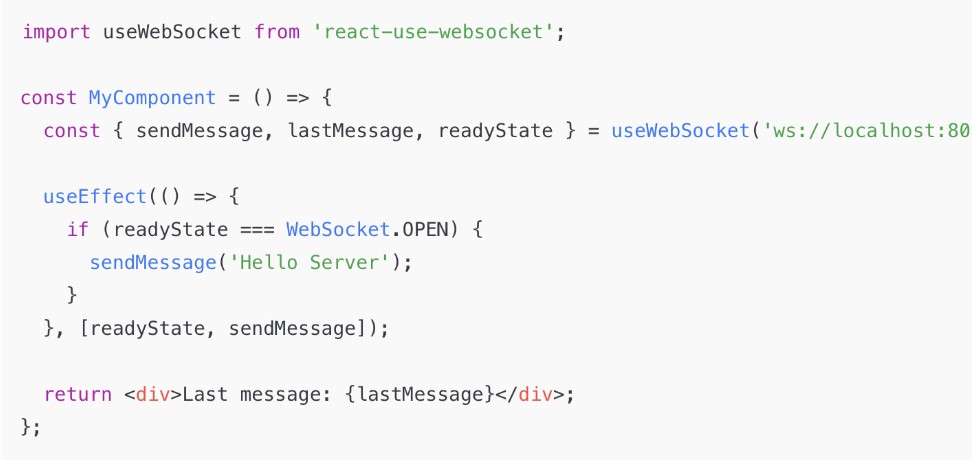
With this hook, you don’t have to stress about managing the WebSocket state on your own. It takes care of the connection and message handling for you, so you can focus on other parts of your app. It makes working with client data super simple, all while ensuring you’ve got a smooth full duplex connection.
If you’re thinking about optimizing your React app further, you might want to explore our guide on what is micro frontend in React.js.
Practical Example: Building a Real-Time Chat Application
Project Overview and Requirements
In this React WebSocket example, we developed a real-time messaging system to support both private and group chats. Users can set up profiles and customize notification settings for an enhanced experience. This required efficient handling of real-time communication, user authentication, and smooth message flow.
Setting Up the Backend Server with WebSockets
The backend was built with NestJS and Socket.IO, using a WebSocket gateway for real-time communication. TypeORM was employed to manage the database, providing an easy way to sync user data and chat messages with minimal overhead. We also integrated authentication, ensuring only authorized users could connect to specific chat channels.
Key Challenges:
- Implementing message broadcasting to multiple users simultaneously.
- Handling reconnections and ensuring message delivery reliability in the case of dropped connections.
Developing the React Frontend
We used React along with Material-UI and TailwindCSS for a responsive and sleek chat interface. The UI was inspired by Telegram, featuring clean layouts and smooth transitions.
Key Features:
- State Management: React’s Context API was used to manage global app state, including chat messages and user sessions.
- UI Components: Components like the message list, user profile, and chat input were built as reusable units for scalability and ease of maintenance.
Key Features and Implementation
- Establishing the WebSocket Connection: Setting up a WebSocket connection in React is very straightforward. If you’re switching from Angular to React, you’ll find that React’s hooks make it easy to manage WebSocket connections—React simplifies the process with its component-based structure. We also handled reconnection logic using Socket.IO’s built-in features, ensuring a stable connection even in the event of network issues.
- Sending and Receiving Messages: Socket.IO enabled real-time message exchange with minimal delay. The client subscribes to messages via events, and the server pushes updates to clients as soon as a new message is received.
Challenges: Ensuring smooth message delivery under high traffic, especially for group chats with multiple active users.
Running and Testing the Chat Application
Testing was conducted mainly on the backend, using end-to-end testing (e2e) to verify communication between the client and server. We focused on ensuring messages were delivered accurately and reliably. The Socket.IO client was tested to ensure it could handle high concurrency and reconnect scenarios.
Need expert help with WebSocket development or React projects? Get in touch with us today to discuss your project and create scalable real-time applications.
Best Practices for WebSockets in React
Handling Reconnection and Connection Loss
WebSocket connections can drop due to network issues or server failures. To prevent your app from breaking, you need a strategy to automatically reconnect. A simple approach is to use an exponential backoff mechanism, which delays reconnection attempts and reduces load on the server. Here’s a practical WebSocket ReactJS implementation:
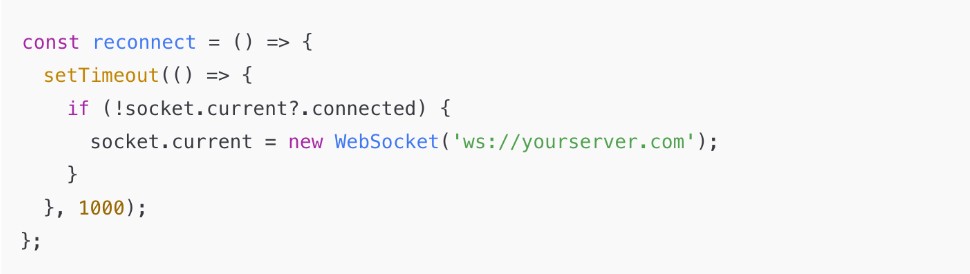
By implementing this reconnection approach, you ensure your app doesn’t stay disconnected for long periods. It also makes the connection more resilient, providing a better user experience, especially when handling callback functions to manage reconnection logic.
Thinking about scaling your app? Keep an eye on the React developer contract rate to plan your budget and timeline effectively.
Optimizing Performance and Resource Management
WebSocket connections consume resources on both the client and server side. Proper cleanup is crucial to prevent memory leaks. When the React WebSocket component is unmounted, ensure that the WebSocket connection is closed to release resources.
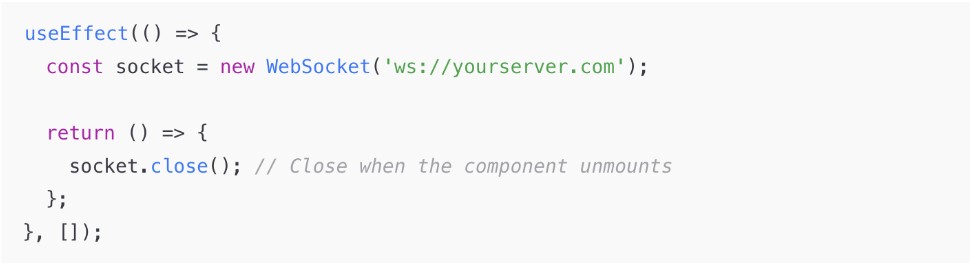
By using useEffect with proper cleanup, you ensure that your app doesn’t leave connections hanging, which significantly saves on resources. It’s a simple way to keep things smooth while working with the WebSocket protocol.
Security Considerations and Secure Connections (WSS)
When using React WebSockets, security is key. Always go for WSS (WebSocket Secure) instead of WS to keep your connection encrypted. This protects sensitive data, such as a login host token or personal info, especially in apps with user authentication or private client data. It’s a simple step to keep your app and users safe.

If you don’t use WSS, data is transmitted in plain text, which makes it vulnerable to man-in-the-middle attacks. To ensure secure communication, React.js alternatives like Next.js or other frameworks can offer additional layers of protection.
Structuring Messages and Using Subprotocols
For more complex WebSocket apps, it’s important to structure messages consistently. JSON is a common format because it’s easy to work with. If your app has multiple message types (e.g., chat or notifications), you can use WebSocket subprotocols and JSON.stringify to send data.

In this example, the WebSocket server knows it’s dealing with a chat application based on the subprotocol (’chat’). The client sends messages as JSON—this allows you to send data in a format that makes it easier for both ends to understand and process the data efficiently.
Advanced WebSocket Techniques
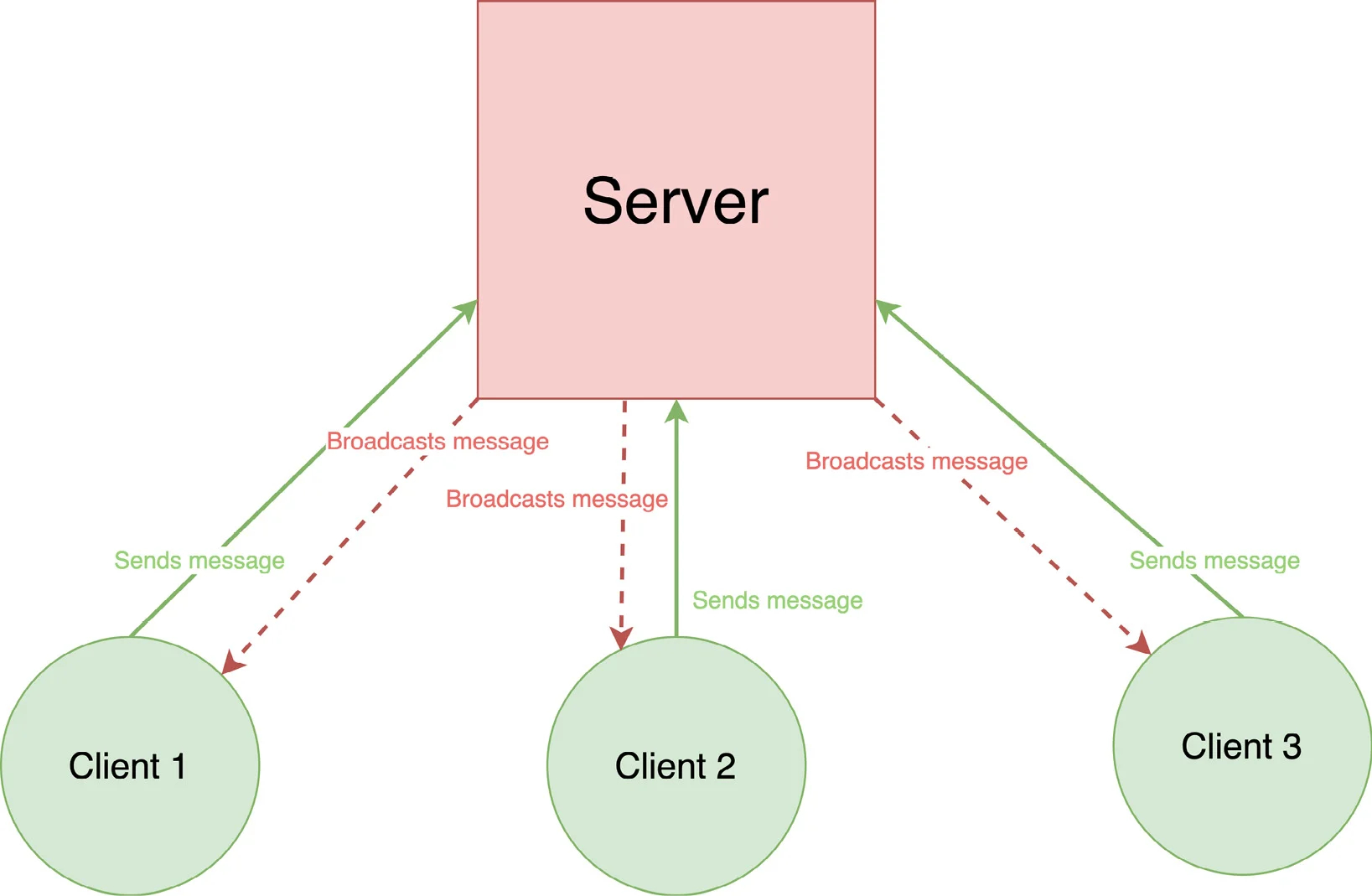
Implementing Heartbeat Mechanisms
One of the challenges with WebSocket connections is ensuring they remain active. Sometimes, connections can be dropped without notice, and this can cause disruptions in your app. To detect inactive clients, implement heartbeat checks by having the server send periodic “ping” messages. The client responds with “pong.” If there’s no response, the server closes the connection and attempts to reconnect.
Broadcasting Messages to Multiple Clients
Sometimes, you need to send the same message to multiple clients at once—maybe you’re building a chat app, a live sports feed, or an auction platform. Use server-side logic to broadcast messages. The server sends one message to all connected clients simultaneously—this reduces the need for individual messages and improves efficiency. Libraries like Socket.IO make this easier by managing group connections.
Scaling WebSocket Applications with Load Balancing
As your app grows, use load balancing to distribute traffic across multiple servers. Scaling WebSockets requires sticky sessions to tie each client connection to the same server. Use tools like NGINX or AWS Application Load Balancer to manage session persistence effectively.
How Next JS is different from React becomes especially clear when moving your React app to Next.js, as it may require extra setup for scaling WebSocket connections, especially for server-side rendering and load balancing. Learn how to move React app to Next.js for improved performance.
Integrating Authentication and Authorization
For apps that require user authentication, ensuring only authorized users can access WebSocket channels is crucial. One way to handle this is by sending a token (like a JWT) when establishing the WebSocket connection.
The server then verifies the token before accepting the connection, rejecting invalid or missing tokens. For more complex setups, you can use role-based access control (RBAC), granting different access levels based on user roles. For example, admins can access all channels, while regular users are limited to specific ones. This process is managed via HTTP request and server-side role checks.
Debugging and Testing WebSocket Applications
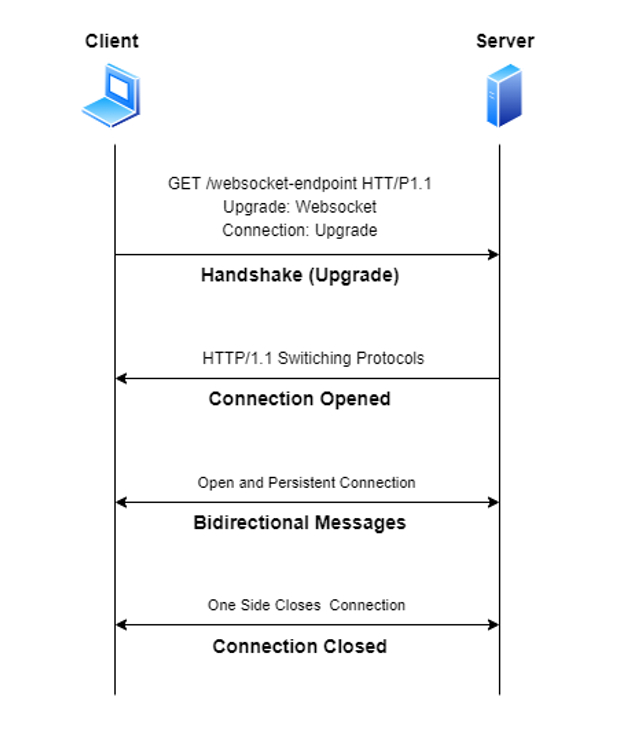
Tools for Monitoring WebSocket Traffic
When you’re building real-time apps with WebSockets, monitoring traffic can help you troubleshoot connection issues or verify message flow. Tools like WebSocket King Client or Wireshark let you capture WebSocket traffic, so you can see the messages exchanged between client and server. These tools also support sending custom WebSocket messages, which is handy for debugging. In React, integrating a React hook for WebSocket monitoring can simplify tracking the state of connections.
If you’re curious about how React and Angular differ in handling real-time communication, take a look at this article on Angular and React.
Common Issues and How to Resolve Them
WebSocket apps are powerful, but they come with their own set of issues. Some common problems include connection drops, message delays, and mismatched data formats. To tackle these, ensure you’re handling events like onclose and onerror properly, and have a HTTP server in place to manage retries and reconnects. For message handling, always verify that you’re sending and receiving data in the correct format, such as JSON.stringify() for sending data and parsing it on the client side.
Writing Unit Tests for WebSocket Logic
Testing WebSocket connections might seem tricky, but it’s totally manageable with the right approach. Use mocking libraries like Jest to simulate WebSocket connections and responses. For example, you can mock the React WebSocket API and test how your app handles incoming received messages or reacts to errors. This ensures that your mobile app or web application behaves correctly without actually needing a live WebSocket server during testing. Writing unit tests will help you catch edge cases and maintain application stability.
Practical Tips for Developing with WebSockets and React
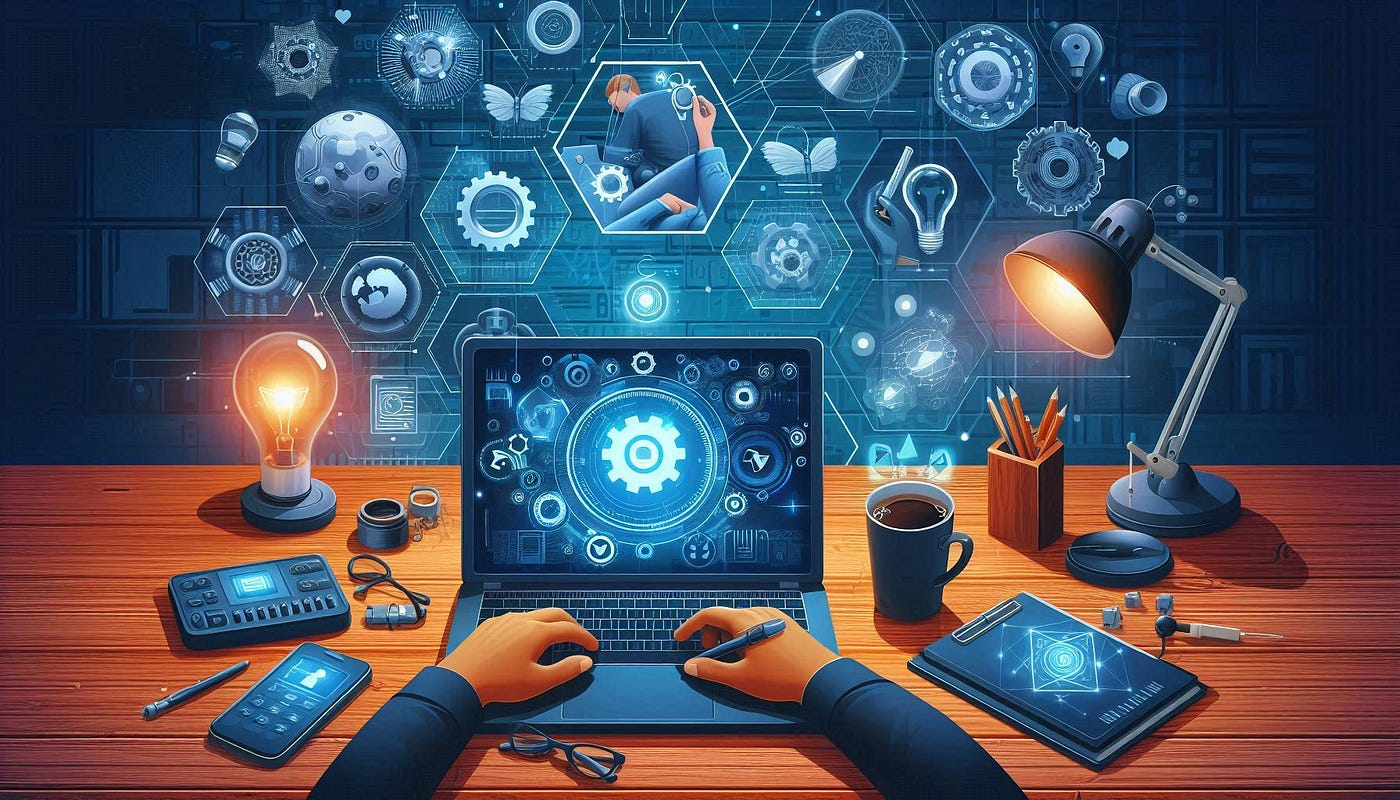
Managing State with Context API or Redux
Use Context API or Redux to manage state in real-time apps. The Context API works for smaller apps, while Redux is better for larger ones. Both tools help avoid prop drilling and keep state in sync with WebSocket data.
Structuring Your Application for Maintainability
Keeping your app organized from the start is key for long-term maintainability. Break your app into smaller, reusable components, and make sure to separate your WebSocket logic into its own module. This way, you’ll avoid cluttering your components with too much responsibility.
Leveraging TypeScript for Better Type Safety
TypeScript ensures type safety, especially with WebSocket data. Define custom types for incoming and outgoing messages to catch errors early and make your code more predictable. This is also useful for working with the HTTP protocol.
Implementing Error Handling and User Notifications
No matter how great your app is, users will eventually encounter errors. Handle errors gracefully by notifying users about connection issues or failed messages. You can also use string options to customize the error messages based on the type of failure, making them more user-friendly.
Conclusion
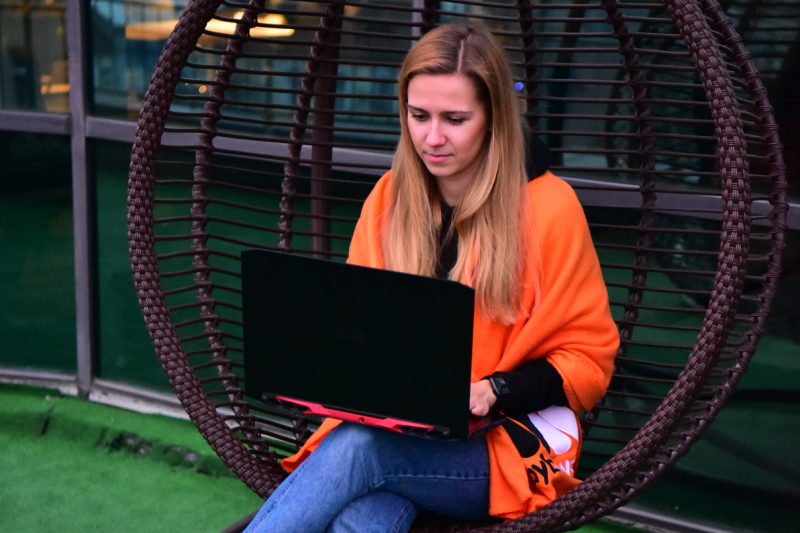
Now that you’ve learned how to implement WebSocket in React, you’re ready to bring real-time interactivity to your apps. Whether you’re building a live chat, a real-time dashboard, or something else entirely, WebSockets allow your app and server to communicate instantly, without refreshing or making constant requests. Keep experimenting and testing your WebSocket connections to ensure smooth performance. For a deeper dive into all the features and advanced use cases, don’t forget to check out the official WebSocket documentation—it’s packed with helpful tips and details to level up your real-time apps!
FAQs
-
Can I use WebSockets with state management libraries like Redux in React?
Yes, WebSockets can work seamlessly with Redux. You can dispatch actions based on WebSocket events and store the data in your Redux store for global access. When you implement WebSocket in React, keep WebSocket logic in middleware like redux-saga or redux-thunk to separate concerns and maintain clean code.
-
What are some common mistakes to avoid when implementing WebSockets in React?
1) Not closing WebSocket connections when a component unmounts, which can lead to memory leaks. 2) Reinitializing connections unnecessarily, especially in components that re-render often. 3) Sending messages before the connection is fully established. Always check the readyState before sending data.
-
How do WebSockets affect the performance and scalability of my application?
WebSockets are lightweight and efficient for real-time communication. However, managing many concurrent connections can strain the server. To scale, use WebSocket servers like Socket.IO or AWS WebSocket API and load balancers designed for persistent connections.
-
How can I manage multiple WebSocket connections within a single React app?
Use a centralized approach, such as a custom hook or context, to manage WebSocket connections. This avoids redundancy and ensures all components share the same connection logic. For example, a custom useWebSocket hook can handle multiple connections by mapping different endpoints to separate WebSocket instances.