Why Follow React Best Practices?
Following React development best practices isn’t just about keeping things tidy—it helps your app grow smoothly and stay manageable. By sticking to good habits like organizing your folder structure and not going overboard with component nesting, you can keep things simple and easy to follow. Plus, focusing on React code readability makes it easier for others (or future you!) to jump in and understand what’s going on.
Here’s why it’s a good idea:
- Easier to scale your app as it grows
- Faster onboarding for new developers
- Fewer bugs and more predictable code
- Better performance and optimization
- Simplified debugging and maintenance
Simply put, following these best practices leads to better, more sustainable React code.
Code Organization and Structure
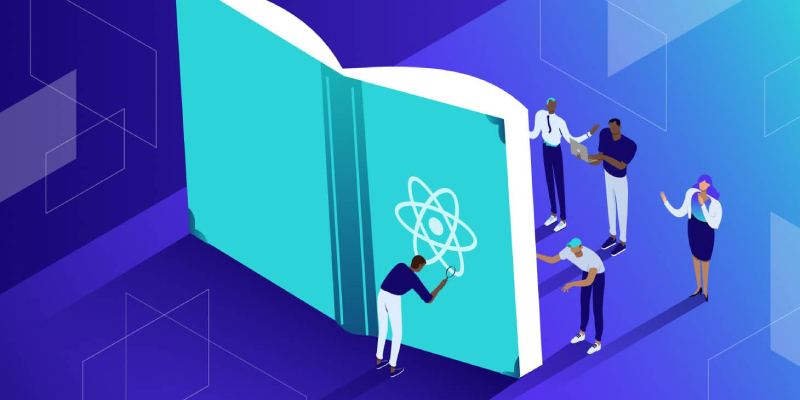
Why it actually matters? Well, good code organization is the backbone of scalable and maintainable React apps. In short, taking the time to organize your code properly now can save a lot of headaches later.
Folder Structure
When it comes to React, how you organize your code can make or break your project. A clean, well-thought-out folder structure keeps everything in the right place and helps your team stay on the same page. Grouping related files together, like components, hooks, and styles, ensures that everything is easy to find when you need it. Plus, it makes scaling your app a lot less painful. Proper organization also plays a role in React.js performance optimization, as it helps ensure that your app is efficient and maintainable as it grows.
Separation of Concerns
A big part of writing maintainable code is keeping your concerns separate. Following React best coding practices, this means keeping your React app UI components focused on rendering and not bogged down by business logic or side effects. Use hooks to manage state and logic, and leave the presentation to your components. This not only keeps your code clean but also makes it easier to test and debug. When each part of your app has a clear responsibility, things stay organized and manageable—so no more chasing down bugs in that one massive file!
State Management Best Practices
State management in React can feel like juggling—you’ve got to keep everything in balance. Whether you’re handling local or global state, it’s important to use the right tools and techniques to keep things under control.
Local State
For small, isolated pieces of data, local state using useState is your go-to. It’s simple and keeps things close to where you need them—ideal for things like form inputs or toggling UI elements. Just remember: don’t overcomplicate things with unnecessary state in deeply nested components.
Global State
When it comes to global state, the best approach is to stick with best practices in React.js: for more complex scenarios, use useReducer or the Context API for efficient management. These tools help you manage state across multiple components without prop drilling, and they provide a cleaner way to handle shared data across your app.
Optimization Techniques
Want to keep your app performing? Consider using React.memo and useMemo to avoid unnecessary re-renders and keep things snappy. Also, look into libraries like Redux or Zustand for more complex state management needs. These options can help optimize performance while maintaining a smooth, scalable app. For more options, explore React alternatives.
Component Design and Reusability
When designing React components, the goal is to keep things modular, reusable, and maintainable. A well-designed component can be easily updated or swapped out without affecting the rest of the app. Here’s how to do it right:
Functional Components
Functional components are key in modern React development. They’re simple to create and easy to manage. Using React component best practices, functional components should focus on a single responsibility—this keeps them clean and easy to test. For example:
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
This is a reusable button component where the props label and onClick are passed from the parent component. The component is small, focused, and easy to update.
That said, class components are still part of React’s history and you’ll likely come across them in older codebases. While most developers now prefer functional components with hooks, it’s still helpful to understand class components, especially when maintaining older projects or learning how React has evolved.
Props and Children
Managing parent-child relationships through props is how data flows in React. Props are passed from parent to child components, but children are a special type of prop that allows components to be more flexible.
For example, a simple card component that accepts children:
function Card({ title, children }) {
return (
<div className="card">;
<h2>{title}</h2>;
<div>{children}</div>;
</div>;
)
}
You can use the Card component and pass any JSX as children:
<Card className="myTitle">;
<p>{This is the content inside the card.}</p>;
</Card>;
This way, the Card component is reusable with any content inside, making it flexible and easy to customize.
Avoiding Prop Drilling
Prop drilling happens when you have to pass props through many layers of components, which can make your code harder to maintain. A better approach is to use React context or useReducer for managing state that needs to be shared across multiple components.
Here’s an example using useContext to avoid prop drilling:
const ThemeContext = React.createContext();
function App() {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>;
<Child />;
</ThemeContext.Provider>;
);
}
function Child() {
return <Grandchild />;
}
function Grandchild() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<dev>;
<p>Current theme: {theme}</p>;
<button onClick={() =
In this example, the Grandchild component can access the theme without the need for prop drilling, making the code much cleaner.
Advanced Patterns
As your React skills grow, you might find yourself needing to implement more advanced patterns like higher-order components (HOCs), render props, or server-side rendering with Next.js.
For example, you may plan to convert React to Next.js to take advantage of server-side rendering (SSR) to improve performance and SEO:
function HomePage() {
return <h1>Welcome to the Home Page</h1>;
}
export async function getServerSideProps() {
// Fetch data server side
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
In this case, the page is rendered on the server before being sent to the client, which helps with faster load times and better SEO. This is a great example of React.js best practices that boosts both performance and scalability. By using SSR, you’re not just speeding things up, but you’re also making sure your React JS applications scale without getting in the way of your project’s growth.
Performance Optimization
React coding best practices aren’t just about writing pretty code—they’re about keeping your app fast, responsive, and scalable. From reducing unnecessary renders to smartly handling side effects, every little tweak counts—whether you’re working on a SPA or a complex enterprise project, these techniques can make all the difference. Let’s dive into some key methods to keep your app running like a well-oiled machine.
![]() CTO’s Expert Advice “At MaybeWorks, we focus on performance optimization from the ground up. By reducing unnecessary renders, using lazy loading, and leveraging React.memo and PureComponent, we ensure smooth scalability. These practices help us minimize technical debt and build high-performance apps that grow with our clients’ needs.” |
Ready to boost your React app’s performance? Let’s chat and make your project future-proof!
Prevent Unnecessary Renders
Unnecessary renders can slow down your app, especially as it grows. To avoid this:
- Use React.memo or PureComponent: These tools ensure components only re-render when their props or state actually change. For example:
const MemoizedComponent = React.memo(MyComponent);
- Optimize state updates: Keep your state updates localized. Avoid lifting state too high unless absolutely necessary, as this can trigger unneeded renders up the component tree.
- Use keys wisely in lists: Proper keys prevent React from unnecessarily re-rendering list items. Avoid using array indices as keys unless the list is static.
Lazy Loading
Lazy loading is your best friend when dealing with large applications. It ensures only the parts of your app that are immediately needed are loaded, improving initial load times.
- Code splitting: Use React.lazy with Suspense to dynamically import components. For instance:
const LazyComponent = React.lazy(() =
- Images and assets: Tools like react-lazy-load-image-component can help you delay the loading of heavy media until they are in view.
- For scalability, consider lazy loading entire routes in a single-page app. This works wonders for performance optimization in apps with many views.
Cleanup Side Effects
Side effects, if not handled correctly, can lead to memory leaks and performance issues. Cleaning up side effects ensures your app stays efficient.
- Use useEffect properly: Always return a cleanup function when setting up side effects like subscriptions or event listeners. For example:
useEffect(() =
- Handle class component lifecycles with care: For legacy code, manage side effects using lifecycle methods like componentDidMount and componentWillUnmount.
- Error boundaries: Use them to catch and handle errors gracefully, preventing entire sections of the app from crashing unexpectedly.
- Monitor data flow: Keeping your app’s stateful logic well-organized prevents unnecessary side effects. For instance, using reusable components within a component-based architecture can isolate functionality and reduce unwanted behaviors.
By implementing these techniques, you’ll improve your app’s responsiveness and lay the groundwork for smoother scaling.
Effective Use of Hooks
Mastering hooks is a cornerstone of modern React development. They simplify code, streamline state management, and unlock reusable logic for complex applications. Whether you’re following React configuration best practices, refining your component design, or fine-tuning your app’s performance, hooks are essential tools in your arsenal.
Rules of Hooks
Hooks come with a set of unbreakable rules to ensure they work as expected:
- Only call hooks at the top level: Never use React hooks inside loops, conditions, or nested functions. For example:
- Only call hooks in React functions: Hooks are exclusive to functional components and custom hooks. Avoid using them outside the React context.
- Follow dependencies carefully: When using useEffect or similar hooks, always include the right dependencies to avoid unexpected behaviors or infinite loops.
// Correct usage
useEffect(() =
Custom Hooks
Custom React hooks are great for abstracting reusable logic, making your code cleaner and easier to maintain. They are perfect for encapsulating functionality that might otherwise clutter your components—an invaluable asset whether you’re handling complex logic or planning to migrate AngularJS to React for a modernized approach.
- Example: A custom hook for fetching data:
import { useState, useEffect} from 'react';
const useFetch = (utl) =
Use it in your components to keep logic modular:
const { data, loading } = useFetch('/api/items');
Common Hooks
Hooks like useState, useEffect, and useContext are the backbone of functional components.
- useState: Manages local state within a component, keeping track of dynamic values like user inputs or UI changes.
- useEffect: For handling side effects like API calls or DOM manipulation. Always remember to clean up after side effects for better performance.
- useContext: To avoid prop drilling by providing a global state to multiple components. It’s great for managing shared data like themes or user authentication.
Testing Best Practices
When building robust React applications, testing acts as your quality assurance toolkit. By following best practices for React, you can catch issues early, improve code reliability, and ensure a smoother user experience. Testing isn’t just about finding bugs—it’s about future-proofing your app and making it easier to maintain as it grows. Polishing your React syntax? Focused on solid standards? Testing makes your app bulletproof.
Unit Testing
Unit testing involves isolating individual components or functions to verify they perform as expected. For example, you might test a button component to ensure it correctly triggers an onClick event or a form validation function to confirm it flags invalid inputs. Tools like Jest and React Testing Library make it simple to write tests that check specific pieces of your application.
Key tip: Focus on predictable outputs. If a function takes A as input and should return B, your test should verify that relationship. This helps ensure code consistency and avoids regressions in future updates.
Integration Testing
Integration tests focus on how different parts of your application work together. For example, you can test how props are passed from parent to child components or ensure that your state management correctly updates the UI when the underlying data changes. Tools like Cypress or Playwright allow you to simulate real-world scenarios, such as navigating between pages or submitting forms.
Key tip: Write tests that mimic actual user interactions. This helps catch issues that unit tests might miss and ensures seamless data flow between your components.
Focus on User Behavior
The ultimate goal of testing is to create an app that behaves as users expect. This means prioritizing tests that simulate real-world interactions, like clicking a button, entering text, or selecting items from a list. Behavioral testing frameworks, such as React Testing Library, emphasize this approach by encouraging tests that mirror user actions rather than implementation details.
Key tip: Aim to test your app from the user’s perspective rather than focusing solely on internal logic. This improves UI components and ensures your application aligns with user needs, enhancing both usability and reliability.
Handling Errors Gracefully
Following React layout best practices is all about your app behaving well under stress and offering users a clear path when errors happen. Let’s dive into how you can handle errors gracefully and ensure smooth sailing for both your users and your team.
Error Boundaries
Error boundaries are one of the most powerful tools React gives you for error handling. They work by wrapping around your components and catching JavaScript errors that occur within their tree, preventing those errors from crashing the entire app. Think of error boundaries as the last line of defense before an issue affects the entire user experience.
When to use error boundaries:
- Critical Components: Wrap components that are crucial to the app’s functionality, such as user authentication or payment processing. If something goes wrong, users can still interact with other parts of the app.
- Third-Party Libraries: Libraries or external APIs can sometimes misbehave. Use error boundaries around components that rely on third-party code to prevent unexpected failures.
- UI Fallbacks: Instead of a blank screen or broken functionality, display a helpful fallback UI to let users know something went wrong, like “Oops! Something went wrong—please try again later.”
Here’s an example:
class ErrorBoundaries extends React.Component {
constructor(props) {
super(props);
this.state = { hasErrors: false };
}
static getDerivedStateFromError(error) {
return { hasErrors: true };
}
componentDidCatch(error, info) {
// Log the error to an error reporting service
logErrorToMyService(error, info);
}
render() {
if (this.state.hasErrors) {
return <h1>Something went wrong!</h1>;
}
return this.props.children;
}
}
Wrap your critical components with <ErrorBoundary> to ensure the rest of the app remains unaffected if an issue occurs.
Monitoring Tools
Just catching errors with error boundaries is great, but proactively monitoring your app’s performance in real-time can save you a lot of time and headaches. Using monitoring tools like Sentry, LogRocket, or New Relic helps you track errors in production and provides you with detailed insights into what went wrong. This is especially important in offshore React development, where distributed teams need to quickly address errors, no matter their location.
Why monitoring tools matter:
- Track real-time errors: You can see issues as they happen, not days or weeks later. This allows your team to act quickly, reducing downtime and improving user experience.
- User session replay: Tools like LogRocket allow you to replay user sessions so you can understand the context around an error. This is incredibly useful for troubleshooting tricky bugs.
- Detailed error reports: These tools capture stack traces, user device details, and network requests, which helps you pinpoint issues faster.
- Integrate with your workflow: You can easily integrate these tools with your project management system (e.g., Jira, GitHub), so your team can manage, prioritize, and fix issues efficiently.
Keeping Dependencies Updated
Keeping your dependencies up-to-date is like making sure your car gets regular check-ups—you don’t want things to break down unexpectedly. In the world of React, outdated dependencies can cause all sorts of problems. When comparing Angular vs. React.js, React’s modular design makes dependency updates simpler, while Angular’s all-in-one framework often involves more extensive adjustments. Here’s how to stay on top of it:
- Use a Dependency Manager (npm or Yarn):
Tools like npm or Yarn help you keep everything organized. They make sure you have the right versions of each package, and they even handle package conflicts for you. Make sure your project has a package-lock.json or yarn.lock file to keep things consistent. - Automate Updates with Tools like Dependabot or Renovate:
Want to skip the manual check-up? Tools like Dependabot or Renovate automatically monitor your dependencies and open pull requests when updates are needed. - Track Breaking Changes:
Not all updates are equal. Some might be minor fixes, while others might introduce breaking changes. So, always check the release notes, especially for major updates—nothing worse than being surprised by a broken feature. - Test After Every Update:
After updating dependencies, make sure you run your tests! It’s the best way to catch anything that might have broken. Automated tests are a lifesaver here. - Watch Out for Security Vulnerabilities:
It’s not just about functionality. Updates also include security patches. Use tools like npm audit or Snyk to make sure your dependencies aren’t vulnerable to exploits. It’s a small step for security, but a giant leap for your app’s safety. - Avoid Dependency Bloat:
Don’t just add libraries because they sound cool. Keep your dependencies lean by removing the ones you don’t actually need. It’ll make your project faster and easier to maintain in the long run.
Dependency Management
Keeping your dependencies in check isn’t just about updating them; it’s about managing them right from the start. By following React project setup best practices, you can ensure your app stays reliable and easy to maintain. Here’s a quick list of steps to help do that:
- Pick Stable, Well-Maintained Libraries:
Always go for libraries with good community support and frequent updates. The more popular the library, the less likely it is to cause problems down the road. - Stick to Semantic Versioning:
Keep an eye on the version numbers. When updating, aim for minor or patch updates (not major ones) unless you’re ready for a full refactor. This helps avoid any surprise changes that might break your code. - Update Regularly:
Set a routine to update your dependencies—every couple of weeks, for example. This prevents your project from falling behind and keeps things running smoothly. - Don’t Overcomplicate:
Sure, there’s a library for everything, but don’t add dependencies just for the sake of it. The more dependencies you add, the harder it is to keep things running smoothly. Keep it simple.
Conclusion
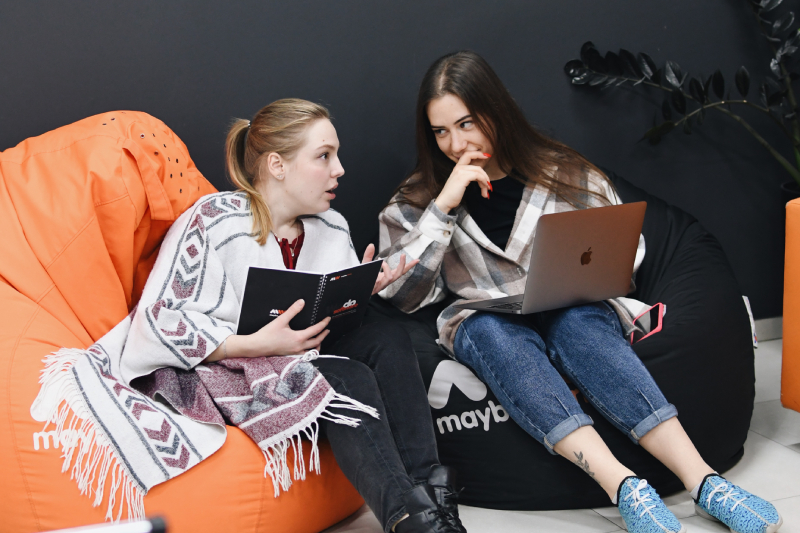
Following React best practices isn’t just about writing good code—it’s about setting your project up for long-term success. By staying organized, optimizing performance, and managing state efficiently, you can build apps that are not only fast but also easy to maintain as they grow. Whether it’s keeping your components reusable or handling side effects the right way, these best practices make a real difference in the quality of your app.
If you want to make sure your React project is heading in the right direction, MaybeWorks has got you covered. Our expert developers can help you apply these best practices, streamline your setup, and ensure your app performs at its best. Get in touch with us today to take your React development to the next level!
FAQ
-
How to structure your React application for better scalability?
Use a component-based architecture, group related files, and follow clear naming conventions. For state management, rely on useState, useReducer, or the Context API for global state. Keep your code organized for easier scaling.
-
When should you use the Context API instead of Redux for state management?
Use the Context API for simple state sharing across components in smaller apps. For complex state with actions and reducers, Redux is a better choice.
-
How to prevent unnecessary re-renders in React components?
Use React.memo or PureComponent to avoid re-renders when props don’t change. Be careful with state and props to avoid passing new references unnecessarily.
-
What is the best way to handle side effects in functional components?
Use useEffect for side effects like fetching data or subscribing to events. Remember to clean up with a return function to avoid memory leaks.
-
How to decide when to create custom hooks?
Create custom hooks to extract and reuse logic across components, like handling forms or data fetching, without cluttering your components.
-
What tools should you use to test React applications effectively?
Use Jest for unit tests and React Testing Library for simulating user interactions and testing component behavior with props and state.
-
How to optimize large lists or tables for better performance in React?
Use React.memo and react-window to render only visible items. useMemo and useCallback can help memoize values and functions to improve performance.
-
How to keep your React dependencies updated without breaking your application?
Use npm-check-updates to track the latest versions and update regularly. Follow semantic versioning and use error boundaries to handle potential issues after updates.