What is React Performance Optimization?
ReactJS performance optimization is all about fine-tuning your React application to ensure it runs smoothly and feels responsive to users. It’s about spotting and fixing those performance hiccups that can slow down your app, making it less efficient and enjoyable to use.
By using the right techniques and strategies, developers can make a big difference in load times, speed up rendering, and generally boost the performance of their React applications. Regularly following these practices and using performance tools provides a clear picture of your app’s performance and helps you make smart, targeted improvements.
Importance of Performance Optimization in React Applications
In today’s competitive digital world, optimizing React performance isn’t just nice to have — it’s a game-changer. Here’s why tuning your app’s performance is critical to its success:
- Better User Experience: A fast, responsive app keeps users happy and engaged, while poor performance can lead to frustration, higher bounce rates, and bad reviews. When your app loads quickly and reacts smoothly, users are more likely to stay with you, explore, and return in the future.
- Higher Conversions: A quick-loading website can directly impact sales and conversions — visitors are more likely to complete actions on a site that doesn’t keep them waiting. Even a slight lag can cause users to abandon their carts or leave before finishing a purchase, so speed directly influences your bottom line.
- Improved Search Engine Rankings: Search engines prefer fast websites, so optimizing your app can help boost your search rankings. Faster load times can lead to better visibility in search results, skyrocketing traffic to your site and giving you a competitive edge.
- Cost Efficiency: An optimized app often uses fewer server resources leading to cost savings. By reducing the strain on your servers, you can lower operational costs and potentially scale your app more efficiently as it grows.
- Stronger Reputation: A well-performing app builds trust and credibility with users. When people know they can rely on your app to be fast and responsive, they’re more likely to recommend it to others, strengthening your brand’s reputation.
React Performance Optimization Techniques
Creating a high-performance React application isn’t just about writing code — it’s about making smart choices throughout development. To ensure the best user experience, developers need to use a combination of strategies and tools. In this section, we’ll explore how to significantly boost your app’s speed, responsiveness, and overall efficiency.
We’ll cover methods like optimizing component rendering, managing state effectively, and pinpointing performance bottlenecks. By understanding and applying these techniques, you can build React applications that perform exceptionally well, even under demanding conditions.
For more insights on ReactJS, including its components, SPAs, and SEO considerations, check out our guide on ReactJS web applications.
Optimizing Component Rendering
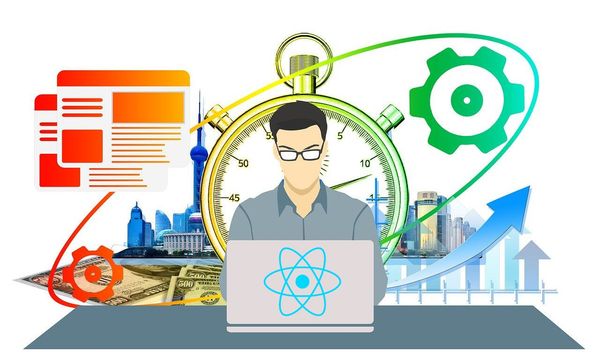
Efficient component rendering is one of the key performance optimization techniques in React. Optimizing how components render is crucial for making your React app both faster and more responsive. One effective technique to achieve this is windowing, which involves rendering only the visible items in a list rather than the entire dataset. This approach can remarkably reduce the number of components that need to be updated and rendered at any given time.
In addition to windowing, implementing techniques like shouldComponentUpdate
, React.memo
, and PureComponent
can help prevent unnecessary updates to your components. This means your app won’t waste valuable time re-rendering parts that haven’t changed, keeping everything running smoothly. Additionally, avoiding inline functions and using useCallback
ensures that functions remain stable across renders, reducing the likelihood of extra updates and making your app feel snappier.
Code-Splitting and Lazy Loading
Code-splitting and lazy loading are further examples of transformative techniques for boosting your React app’s performance. Using React.lazy
and Suspense
, you can load components only when they are actually needed, which helps your app start up faster since it doesn’t have to load everything all at once.
Dynamic imports facilitate this by breaking your code into smaller, manageable chunks that are loaded on demand, which enhances the speed and responsiveness of your app. Additionally, lazy loading route components and optimizing your bundle size contribute to reduced load times. Techniques like prefetching and preloading ensure that crucial resources are prepared and available as soon as needed, leading to a smoother and more efficient user experience overall.
Memoization Techniques
Memoization techniques are essential for enhancing React performance optimization tools as they reduce unnecessary computations and re-renders. By caching the results of expensive calculations, these techniques improve performance and efficiency. For example, the useMemo
hook allows you to cache the results of functions and only recompute them when their dependencies change, preventing redundant calculations.
Similarly, React.memo
memoizes functional components to avoid unnecessary re-renders when props haven’t changed. Additionally, avoiding inline functions helps maintain stable references, reducing the chances of extra re-renders and ensuring smoother performance. Implementing these strategies can make your application faster and more responsive, enriching the overall user engagement. For additional insights into how to optimize performance in React, consider exploring options to hire ReactJS developers.
Optimizing List Rendering
Optimizing list rendering is key to enhancing React performance, especially when working with big data. By using techniques like adding key props to each list item, you help React keep track of changes more efficiently, which speeds up updates and rendering.
Another powerful approach is virtualization, which only renders the items currently visible on the screen using libraries like react-window
or react-virtualized
. This reduces the number of elements in the DOM, making your app feel much faster. These strategies ensure that your list-based interfaces are smooth and responsive, providing a better experience for your users.
Efficient State Management
Efficient state management is vital for optimizing React application performance and ensuring your JS app runs smoothly. Good state management helps prevent unnecessary re-renders and ensures your app remains responsive. Tools like React’s Context API, Redux, or Recoil offer structured ways to manage and update state, making it easier to handle complex interactions.
Techniques such as batching state updates and memoizing selectors can further reduce your app’s workload, keeping things fast and efficient. By focusing on smart state management, you enhance your app’s performance and deliver a seamless UX. For more on React’s capabilities, take a look at our guide on React scope.
Reducing Bundle Size
Reducing bundle size is key to making your React app faster and more responsive. A smaller bundle means quicker load times, which is crucial for keeping users engaged. You can cut down on bundle size by using techniques like tree-shaking to remove unused code and code-splitting to break your app into smaller, manageable chunks that load only when needed.
Minification and compression further reduce the size of your JavaScript files. Tools like Webpack and Rollup are great for managing this process and ensuring you include only what’s necessary. By focusing on bundle size, you’re improving React performance and making your app not only faster but also more efficient.
Using Developer Tools for Performance Monitoring
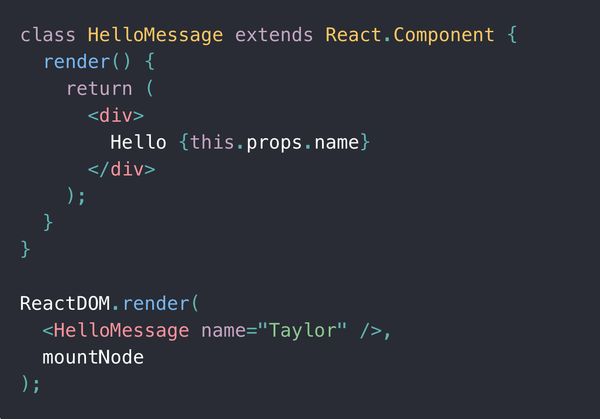
Using developer tools for performance monitoring is essential for understanding and improving how to optimize performance in React. Tools like React DevTools and Chrome DevTools offer insights into your app’s performance, helping you spot issues and inefficiencies. React DevTools is a fantastic resource for understanding your app’s performance at the component level. It lets you see how your components are rendering and how state changes are affecting them. The Profiler tab is especially useful for tracking how long each component takes to render, which helps you identify and address any performance hiccups.
On the broader side, Chrome DevTools offers a suite of features for a more in-depth performance check. With the Performance panel, you can record and analyze your app’s runtime, see detailed information on CPU usage, and catch any JavaScript performance issues. The Memory panel is great for spotting and fixing memory leaks, while the Network panel helps you keep tabs on how quickly resources are loading, which is crucial for optimizing load times.
How Can MaybeWorks Help You Optimize React Performance on Your Project?
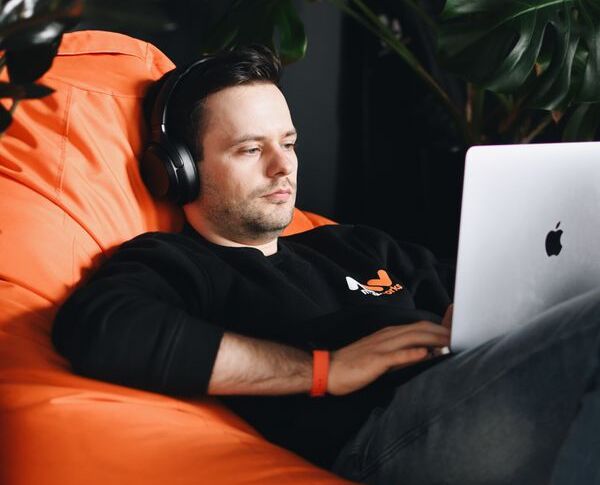
MaybeWorks can make a big difference in improving ReactJS performance for your project. We know how crucial it is to have a fast and responsive application, so we focus on practical strategies that boost performance. Our team is skilled in areas like optimizing component rendering, managing state efficiently, and implementing code-splitting and lazy loading to keep your app running smoothly.
We start by diving into your app to spot any performance issues and figure out the best ways to fix them. With tools like React DevTools and Chrome DevTools, we get a clear picture of how your app is performing and make informed adjustments to enhance speed and efficiency.
If you’re considering AngularJS to React migration, we’ve got you covered there too. We’ll guide you through the transition, ensuring that your new React app makes the most features like the virtual DOM for optimal performance. At MaybeWorks, our goal is to help you create a smarter app that delivers an excellent user experience. Find out how we can help by reaching out today and see for yourself how our expertise can make a difference.
Conclusion
Optimizing React performance is crucial for ensuring your app is fast, responsive, and enjoyable for users. By focusing on techniques like efficient component rendering, code-splitting, lazy loading, and memoization, you can significantly boost your app’s speed and responsiveness. Tools like React DevTools and Chrome DevTools are invaluable for monitoring performance and spotting issues that need fixing.
Whether you’re refining an existing project or moving from AngularJS to React, using these optimization strategies will lead to a smoother, more reliable application. At MaybeWorks, we can help you import these techniques into your development process. Reach out today to see how we can boost your React app’s performance and offer a superior user experience.
FAQs
-
What is code-splitting, and how does it improve application performance?
Code-splitting divides your application into smaller chunks, which are loaded on demand. This reduces initial load time and helps optimize React app performance, especially for large applications.
-
What are some strategies for reducing the bundle size of a React application?
Optimize images, minimize code (tree-shaking, minification), use lazy loading, remove unused dependencies, and leverage code splitting.
-
What tools can be used to monitor and profile the performance of React components?
React DevTools, Chrome DevTools, and dedicated performance profiling tools like Lighthouse can be used to identify performance bottlenecks.
-
What are some common mistakes developers make that lead to poor performance in React apps?
Inefficient rendering (avoiding unnecessary re-renders), large component trees, excessive state updates, and ignoring performance budgets are common culprits. Understanding how to optimize performance in React can help avoid these issues.
-
How can dynamic imports help optimize a React application’s performance?
Dynamic imports load code on demand, improving initial load time and reducing bundle size. This is especially useful for large components or code that are not immediately needed.
-
How does the profiling feature in React DevTools assist in performance optimization?
It helps identify slow components, measure rendering times, and visualize component hierarchies, aiding in pinpointing performance issues.
-
What is the benefit of using virtualized lists with React Virtualized or React Window?
Virtualized lists render only the visible items, significantly optimizing performance in React for large lists by avoiding rendering unnecessary components.